We first mentioned PHP required fields when discussing PHP forms. It means that some of the input fields in a form must be filled if users wish to proceed with the form submission process.
A little asterisk (*) is commonly used to mark the required fields. For example, if you are registering to a new online shopping website, you might have to provide your address so the ordered goods would come to you.
In this tutorial, we will explain how to set required input fields instead of optional ones. It will help you manage the data that users input during registration or commenting. PHP required fields might also be programmed to display error messages when left empty.
Contents
PHP Required Field: Main Tips
- Using PHP forms you can set certain fields to be required and display error messages if they aren't filled in upon submission.
- This is essential when making any form for actual use with databases and validation.
Example of a Form
Here's a list of fields we are going to use in our example form:
- Fullname: required, must only contain letters and spaces.
- Email: also required, must be properly formatted.
- Website: optional. If present, must be properly formatted.
- Feedback: optional, the text area allows to enter multiple lines.
- Gender: required, one of the options must be selected.
In the following code we have a few specific variables. We will use them to display errors in case required fields are left empty: $name_error
, $email_error
, $gender_error
, and $website_error
.
This can be achieved by using if else
conditionals for every $_POST
variable. These statements PHP check if variable is empty using the empty()
function. In case it is, we store an error message inside the error variable.
Assuming the PHP required fields are all filled in as intended, we continue with our proc_input()
function to validate the input:
<?php
$fullname_error = $email_error = $gender_error = $website_error = "";
$fullname = $email = $gender = $feedback = $website = "";
if ($_SERVER["REQUEST_METHOD"] == "POST") {
if (empty($_POST["fullname"])) {
$fullname_error = "Required";
} else {
$fullname = proc_input($_POST["fullname"]);
}
if (empty($_POST["email"])) {
$email_error = "Required";
} else {
$email = proc_input($_POST["email"]);
}
if (empty($_POST["website"])) {
$website = "";
} else {
$website = proc_input($_POST["website"]);
}
if (empty($_POST["feedback"])) {
$feedback = "";
} else {
$feedback = proc_input($_POST["feedback"]);
}
if (empty($_POST["gender"])) {
$gender_error = "Required";
} else {
$gender = proc_input($_POST["gender"]);
}
}
?>
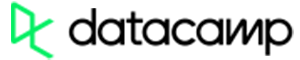
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
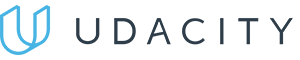
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
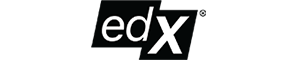
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Displaying Error Messages
Finally, we add a bit of code next to each of the PHP required field in the HTML code. It is meant to display the error messages we have set up:
<form method="post" action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]);?>">
<input type="text" name="fullname" placeholder="Full name">
<span>*<?php echo $fullname_error;?></span><br>
<input type="text" name="email" placeholder="E-mail">
<span>*<?php echo $email_error;?></span><br>
<input type="text" name="website" placeholder="Website URL">
<span><?php echo $website_error;?></span><br>
<textarea name="feedback" rows="6" cols="50" placeholder="Feedback"></textarea><br><br>
<input type="radio" name="gender" value="f">F
<input type="radio" name="gender" value="m">M
<span>*<?php echo $gender_error;?></span><br><br>
<input type="submit" name="submit" value="Submit">
</form>
PHP Required Field: Summary
- If you set certain fields in the forms as required and they are left empty, an error message will be outputted.
- Required fields ensure you get all the data you need from users during registration (or other process).