PHP echo and print statements are language constructs and not functions. You are free to use them without parentheses.
Both of these constructs are for outputting strings. Differences between print and echo constructs are minor but echo can be seen as a more time-efficient option.
Additionally, echo can accept several parameters, while print can be used as an expression since it can return a value of 1.
Contents
PHP echo and print: Main Tips
- PHP
print
andecho
statements are one of the most commonly used constructs to output data (text, variables, etc.). - You don't need to use parentheses with them since they are technically not functions.
print_r
is a function, mostly used for debugging.
echo Language Construct: Proper Use
By using PHP echo
, you can print one or multiple strings. Let's look at a PHP example of how you can achieve that:
<?php
echo "<h2>Am I ready?!</h2>";
echo "Howdy!<br>";
echo "I'm ready!<br>";
echo "I ", "made ", "this ", "string ", "with my handy hands!";
?>
In the code below, you can learn how to display both text and values of variable values by using echo
. You can see 4 different variables in this PHP example: $text1
, $text2
, $var1
and $var2
:
<?php
$text1 = "I am ";
$text2 = "Ready!";
$var1 = 4;
$var2 = 3;
echo "<h2>" . $text1 . $text2 . "</h2>";
echo $var1 + $var2;
?>
Note: when you assign parameters to echo, you need to write them within parentheses.
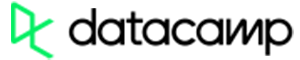
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
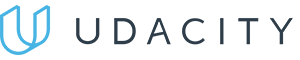
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
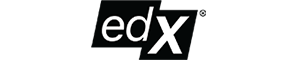
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
How print Is Used
This PHP example below shows how you can display text using PHP print
and include HTML markup into the statement:
<?php
print "<h2>I am ready to learn PHP!</h2>";
print "Hey there!<br>";
print "I will learn ALL the PHP!";
?>
The code of the PHP example below shows how you can display both text and a PHP variable using PHP print
. Again, you can see four variables being used and their values outputted:
<?php
$text1 = "Learning PHP";
$text2 = "ALL the PHP";
$var1 = 58;
$var2 = 4;
print "<h6>" . $text1 . $text2 . "</h6>";
print $var1 + $var2;
?>
How print_r Is Different from print and echo
The use cases of echo
, print
and print_r
in PHP are different.
echo
and print
output strings. print_r
in PHP returns details about variables in a more human-readable form. It provides data type of variables. When used on arrays, print_r
returns all the elements in the list.
In this code example, we are making PHP print arrays by using the print_r
function:
<?php
$a = array ('a' => 'airplane', 'b' => 'train', 'c' => array ('o', 'k', 'f'));
print_r ($a);
?>
Note: to show information in multiple lines, apply \n with the print_r. Also, include <pre> to indicate several lines.
PHP echo and print: Summary
print
andecho
PHP statements are for displaying text or variables.- Parentheses are not required with these language constructs.
print
andecho
output information to the screen, whileprint_r
in PHP shows information about variables in a more human-readable way.