We've already learned to open and read various external files, but sometimes you have to straight-up upload files to server. PHP has useful built-in functions for that.
During the PHP file upload process, set limits to what type of files are allowed in your code, and determine when files are too big.
Everything your code holds might be called $_FILES. This superglobal variable is an array that contains all the data on the files you upload.
Contents
PHP File Upload: Main Tips
- PHP file upload allows the user to upload text and binary files.
- When you use PHP, upload files process requires a permission.
- To upload files in a specific way, you can set up limitations.
- The associative array of all the files uploaded to the script is called PHP
$_files
.
Configuring php.ini
If we wish to make PHP upload files, we have to have the permission. If we don't, we should set up PHP to allow it. To achieve that, we edit the file called php.ini and change the value of file_uploads
to On
as the example shows:
file_uploads = On
Creating HTML Form
After we prepare the permission to initiate PHP file upload, we can get to creating an HTML form for the uploading process:
<!DOCTYPE html>
<html>
<body>
<form action="upload.php" method="post"enctype="multipart/form-data">
Select the image you want to upload:
<input type="file" name="file_to_upload"id="file_to_upload">
<input type="submit" value="image upload"name="submit">
</form>
</body>
</html>
To upload PHP files using these forms effectively, remember a few things:
- When uploading files via HTML forms, we must use
post
method which we assign to the form element as an attribute. - We must also assign
enctype="multipart/form-data"
among its attributes. This allows the browser to identify the encoding type in order to make PHP upload files correctly.
Note: the attribute type="file" of the <input> element displays the input field as a file-select control next to a Browse button.
Uploading Script
The code of file uploading process is held in the upload.php file:
<?php
$target_dir = "uploads/";
$target_file = $target_dir . basename($_FILES["file_to_upload"]["name"]);
$upload_ok = 1;
$image_file_type = pathinfo($target_file,PATHINFO_EXTENSION);
// checking if image file is accessible
if(isset($_POST["submit"])) {
$check = getimagesize($_FILES["file_to_upload"]["tmp_name"]);
if($check !== false) {
echo "The file you picked is an image - " . $check["mime"] . ".";
$upload_ok = 1;
} else {
echo "The file you picked is not image.";
$upload_ok = 0;
}
}
?>
Let's break the example down and make sure we understand every step:
$target_dir = "uploads/"
tells the server where to put the uploaded file.$_files
PHP is the array that holds files uploaded to the code usingPOST
method.$target_file
declares the path to the file you wish to upload.$upload_ok=1
won't be used immediately. It determines whether the upload was successful.$image_file_type
keeps the file extension.- Lastly, we must check if the file is valid.
Note: a new directory called uploads must be created in the path where the upload.php file is placed. The files that are uploaded will be placed there.
Checking If a File Already Exists
In this step, we will add limitations to the form. First, check whether the file we're uploading is not already in our uploads' directory. If it is, $upload_ok
is set to 0
:
// checking whether the file is already present
if (file_exists($target_file)) {
echo "File already present.";
$upload_ok = 0;
}
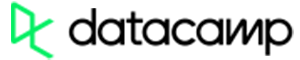
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
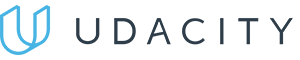
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
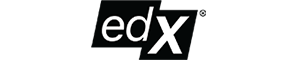
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Setting Up Limits
File Size
We declared a field called file_to_upload
in our form. Now, we should check the file size. If the file size exceeds 500 kylobytes (which is the value we set up), an error is triggered. $upload_ok
will then be set to 0:
// Checking file size
if ($_FILES["file_to_upload"]["size"] > 500000) {
echo "File too big.";
$upload_ok = 0;
}
File Type
Let's look at the example below. You will notice it limits PHP file uploading to only certain types of files. The ones that are allowed must have a JPG, JPEG, PNG, or GIF extension.
If the file does not match the specified types, an error is caught and $upload_ok
is set to 0:
// Limiting what file formats are allowed
if($image_file_type != "gif" && $image_file_type != "jpg" && $image_file_type != "jpeg" && $image_file_type != "png") {
echo "Only JPG, JPEG, PNG & GIF files are allowed.";
$upload_ok = 0;
}
Script of a Successful Upload Process
This is how the final result of making PHP upload files looks:
<?php
$target_dir = "uploads/";
$target_file = $target_dir . basename($_FILES["file_to_upload"]["name"]);
$uploadOk = 1;
$image_file_type = pathinfo($target_file,PATHINFO_EXTENSION);
// Check if image file is a actual image or fake image
if(isset($_POST["submit"])) {
$check = getimagesize($_FILES["file_to_upload"]["tmp_name"]);
if($check !== false) {
echo "The file you picked is an image - " . $check["mime"] . ".";
$upload_ok = 1;
} else {
echo "The file you picked is not an image.";
$upload_ok = 0;
}
}
// Check if file already exists
if (file_exists($target_file)) {
echo "File already present.";
$upload_ok = 0;
}
// Check file size
if ($_FILES["file_to_upload"]["size"] > 500000) {
echo "File too big.";
$upload_ok = 0;
}
// Limit allowed file formats
if($image_file_type != "jpg" && $image_file_type != "png" && $image_file_type != "jpeg" && $image_file_type != "gif" ) {
echo "Only JPG, JPEG, PNG & GIF files are allowed.";
$upload_ok = 0;
}
// Check if $upload_ok is set to 0 by an error
if ($upload_ok == 0) {
echo "Your file was not uploaded.";
// If all the checks are passed, file is uploaded
} else {
if (move_uploaded_file($_FILES["file_to_upload"]["tmp_name"], $target_file)) {
echo "The file ". basename( $_FILES["file_to_upload"]["name"]). " was uploaded.";
} else {
echo "A error has occured uploading.";
}
}
?>
PHP File Upload: Summary
- You can upload text and binary files to your script.
- If you wish to limit uploading files, you can set up certain boundaries.
- Files uploaded to your PHP code are contained in an associative array called PHP
$_files
.