Contents
PHP Array Length: Main Tips
- This function is used to get the number of elements in an array (in other words, to get PHP array length).
- Introduced in PHP 4, though
mode
parameter used for multidimensional arrays only appeared in PHP 4.2.
count() Function
To get you started, we'll view a simple example. In it, we have an array called $fruits
. Using PHP count()
function, we will be able to easily count the elements in contains, or define PHP array length:
<?php
$fruits = ['Banana', 'Cherry', 'Apple'];
echo "Result: " . count($fruits);
?>
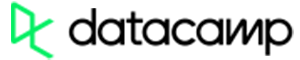
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
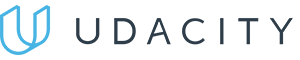
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
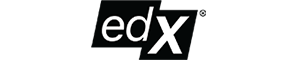
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Learn Proper Syntax
If we wish our PHP count()
function to work and let us get PHP array length easily, we should follow the syntax described below:
count(array, mode);
The parameters are explained in more detail in the table that follows:
Parameter | Description |
---|---|
array | Necessary. Specifies the array to count. |
mode | Optional. Specifies PHP array length counting mode: 0 - Default. Only counts elements in a particular array. 1 - Counts elements in all arrays a multidimensional array contains. |
Counting Examples
The example we have here is meant to show you the difference between different PHP array size counting modes. In it, $fruits
is a multidimensional array that contains three arrays that have seven elements between them. Using different modes fetches us different PHP array sizes:
<?php
$fruit = [
'Banana' => ['Green', 'Yellow'],
'Apple' => ['Green', 'Yellow', 'Red'],
'Berry' => ['Blueberry', 'Strawberry']
];
echo "Normal count: " . count($fruit)."<br>";
echo "Multidimensional count: " . count($fruit, 1);
?>
Let's see a few more examples of getting PHP array length using count()
:
<?php
$fruit = [
'Banana' => ['Green', 'Yellow'],
'Apple' => ['Green', 'Yellow', 'Red'],
'Berry' => ['Blueberry', 'Strawberry']
];
echo "Normal count: " . count($fruit)."<br>";
echo "Multidimensional count: " . count($fruit, 1);
?>
<?php
$fruits = ['Banana', 'Cherry', 'Peach'];
$array_length = count($fruits);
echo "Result: " . $array_length;
?>