One of the most convenient ways to search for specific data is AJAX search box. It is also called live search because it reacts to the users' input.
When users are typing, the live search shows suggestions on how to complete the keyword. It might be enough to enter one character for the box to autocomplete. To create such a search engine, you have to combine AJAX and PHP. We show this process in the following article.
Contents
AJAX Search: Main Tips
- By using AJAX and PHP, you can create a live search web application.
- Users prefer live search because of its speed and suggestions provided as they type.
- You will find an example of a simple AJAX PHP search application below. Results in the example are taken from this example.
Our Search Options
To make the example simple and easy to understand, we will limit our search to three possible values. They will be the same ones we used in the AJAX form tutorial:
<form>
<select name="users" onchange="showUser(this.value)">
<option value="">Select a person:</option>
<option value="johny">Johny Dawkins</option>
<option value="margaret">Margaret Johnson</option>
<option value="julie">Julie Doodley</option>
</select>
</form>
Let's see how this live search will work. Enter any character to the input field. If any of our results contain this character, you will see it as a suggestion:
We will also see and analyze the code needed to make it work. We will start with an HTML file, and then see the PHP file in which the search executes.
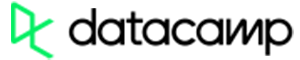
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
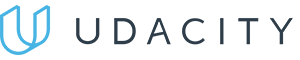
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
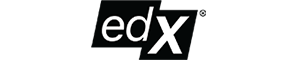
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
HTML Code for Live Search
Upon writing a character into the input text field, we call the function show_result()
. This function will be triggered by the onkeyup
event.
Take a look at this code example, showing how to create a live search for your website:
<html>
<head>
<script>
function show_result(str) {
if (str.length==0) {
document.getElementById("live_search").innerHTML="";
document.getElementById("live_search").style.border="0px";
return;
}
if (window.XMLHttpRequest) {
// script for browser version above IE 7 and the other popular browsers (newer browsers)
xmlhttpreq=new XMLHttpRequest();
} else {
// script for the IE 5 and 6 browsers (older versions)
xmlhttpreq=new ActiveXObject("Microsoft.XMLHTTP");
}
xmlhttpreq.onreadystatechange=function() {
//check if server response is ready
if (this.readyState==4 && this.status==200) {
document.getElementById("live_search").innerHTML=this.responseText;
document.getElementById("live_search").style.border="1px solid #A5ACB2";
}
}
xmlhttpreq.open("GET","/learn/livesearch.php?q="+str,true);
xmlhttpreq.send();
}
</script>
</head>
<body>
<form>
<input type="text" size="30" onkeyup="show_result(this.value)">
<div id="live_search"></div>
</form>
</body>
</html>
If the text input field is left absolutely empty (no characters - str.length==0
), the content of the live_search
placeholder is cleared by the function and the function is terminated.
If there are characters in the input field, then show_result()
function makes an XMLHttpRequest object. The created function will then be executed once the server response is ready.
livesearch.php: Search File
Javascript in the HTML form calls for the file livesearch.php in the server. The script inside it searches for any title that matches the search string. When it's done, the script returns the result:
<?php
$xml_doc = new DOMDocument();
$xml_doc->load('persons.xml');
$x=$xml_doc->getElementsByTagName('name');
$q = $_GET['q'];
$result = '';
foreach($x as $node) {
if (stripos("{$node->nodeValue}", $q) !== false) {
$result .= "{$node->nodeValue}";
}
}
// Set $response to "No records found." in case no hint was found
// or the values of the matching values
if ($result == '')
$result = 'No records found.';
//show the results or "No records found."
echo $result;
?>
If during the AJAX PHP search Javascript sends any text, this happens:
- An XML document is loaded in an XML DOM object.
- The script loops through every element to find a match for the needed text.
- If one or more matches are found, they are returned to display.
- In case no matches are present,
$response
is set to"No records found."
.
AJAX Search: Summary
- Creating live search applications requires combining AJAX and PHP.
- A lot of users prefer live searches to traditional ones because of its speed and useful suggestions.