After you learn PHP basics, you will see dollar signs not only in your dreams but also on your screen, as this symbol ($) is used to mark variables. A PHP variable is similar to a little container that is used to store information (value). A value usually consists of letters or numbers, but a variable can also exist without storing any value.
You are not required to declare a variable before adding a value to it. The first time you assign a value to a particular variable name, you create a variable. The value is assigned by using the equal sign, also called the assignment operator (=). Repetition of the process with the same name results in a change of value.
PHP will also automatically understand the type of the variable according to the value you have assigned to it. The context in which a particular PHP variable works is called PHP variable scope.
Contents
PHP Variable: Main Tips
- PHP variables start with a $ sign.
- PHP variables are used to store information (value).
- The name of a variable can begin with a letter or an underscore character (_), but never a number. However, it may contain numbers.
- Variables are case-sensitive.
What a Variable Is
A PHP variable may have a name as long or short as you wish. It can consist of one letter (x, y, z, and so on) or a whole bunch of them (tree, household, thelongestwordinthewholewideworld).
The example below shows that the variable $text
is a container to the value of Hey coders!
and both $x
and $y
variables hold their respective values of 9
and 1.
<?php
$text = "Hey coders!";
$x = 9;
$y = 1;
?>
Note: In order to assign text to a variable correctly, quotes are used. They are not needed for numerical values.
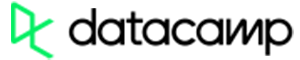
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
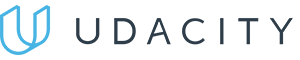
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
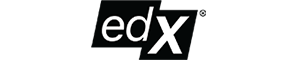
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Way to Output
If you wish to output the information to the screen, it's easy to do by using an echo statement. In the example below, you can see a PHP variable being used in the echo
statement. The result will be the output of both statement text and the value that has been assigned to the $website
variable.
<?php
$website = "bitdegree.org";
echo "I want to visit $website!";
?>
The Scope
The user can declare variables anywhere in the PHP script, outside of a function or within one. The exact part in which a certain variable can be used is called PHP variable scope. There are three main scopes:
- Local
- Global
- Static
Local vs. Global
By expressing a PHP variable outside the function, you make it a PHP Global variable. This means that a certain variable can be used anywhere outside the function.
<?php
$x = 10; // global scope
function learnTest() {
// using x inside this function will generate an error
echo "The x inside function is: $x";
}
learnTest();
echo "The x outside function is: $x";
?>
If you express a variable inside the function, it gives it a Local variable scope. As the name suggests, this PHP variable will be only usable locally: inside that particular function.
<?php
function learnTest() {
$x = 9; // local scope
echo "Variable x inside function is: $x";
}
learnTest();
// using x outside the function will generate an error
echo "Variable x outside function is: $x";
?>
Note: Different variables with local PHP variable scopes can have identical names and still be executed correctly if they are used within different functions.
Global
If you wish to use a PHP global variable inside a certain function, you should use the keyword global
in front of the variable. In the example below you can see how PHP variables $x
and $y
are used inside a function called learnTest()
.
<?php
$x = 10;
$y = 10;
function learnTest() {
global $x, $y;
$y = $x + $y;
}
learnTest();
echo $y; // outputs 20
?>
Global variables are stored in a $GLOBALS[index]
array. These variables can be accessed and updated without leaving the function. This example illustrates how it works in the learnTest()
function:
<?php
$x = 20;
$y = 10;
function learnTest() {
$GLOBALS['y'] = $GLOBALS['x'] + $GLOBALS['y'];
}
learnTest();
echo $y; // outputs 30
?>
Static
A local PHP variable scope also indicates that after a certain function is done, the variables inside are deleted. Sometimes, we might prefer to keep them longer.
For a local variable to stay after the function is executed, a static
keyword must be used when declaring it. You can see an example of how it is applied on the PHP variable $x
below.
<?php
function learnTest() {
static $x = 0;
echo $x;
$x++;
}
learnTest();
learnTest();
?>
The variable will keep both its local scope and the data it held before. It will not be deleted, no matter how many times you will repeat the function.
PHP Variable: Summary
- You can recognize a PHP variable from a first glance, as it always starts with a $. While it's name can contain numbers, it cannot start with one: the first symbol must be either a letter or an underscore (_).
- Information stored in a certain PHP variable is called value.
- Unlike functions, variables are case-sensitive.