In our previous tutorial, we learned about eight different data types used in coding. PHP string is one of the simplest types. Basically, it is a series of characters (letters, numbers, or special characters).
PHP string length can vary from one character to infinity, but remember that all of them have to be surrounded by quotes to be processed correctly. For example, "120" is a simple example of a string. However, if you forget quotes and write the string as 120, it will be read as an integer.
Strings can be used to declare values of variables, or together with echo or print statements to output the characters it holds. PHP has a whole bunch of inbuilt functions meant for handling data strings. We will review five most common ones.
Contents
PHP String: Main Tips
- A string is a character sequence, for instance, "Hello World!". The number of those characters is called PHP length of string.
- Strings are one of the eight PHP data types.
- PHP has inbuilt functions for processing strings.
Useful Functions Explained
In this tutorial, we will introduce you to the most commonly used PHP string functions. They come in handy when you need to fetch PHP string length quickly, have a certain PHP string replaced or searched. To view a more extensive list of functions you can use to handle strings, see our cheat sheet.
strlen()
This function returns the amount of characters in the specified string as a number (in other words, gives you PHP length of string):
<?php
$str1 = 'Hey Ben!';
echo 'The length of the string above is ' . strlen($str1);
// returns 8 and then outputs it
?>
str_word_count()
This function returns the amount of individual words in the specified PHP string as a number:
<?php
$mystring = "Hey Ben, fancy seeing you here!";
print_r(str_word_count($mystring, 1));
?>
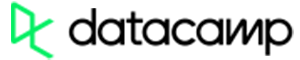
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
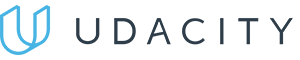
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
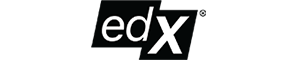
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
strrev()
This function returns the string in reverse. By using this function on a string "Hello World!"
, you would receive "!dlroW olleH"
:
strpos()
This function searches a PHP string for a match of text. After finding the match, it returns the position of the first occurence as a number:
<?php
function look($search, $string){
$position = strpos($string, $search, 5);
if ($position == true){
return "Found: position " . $position;
}
else{
return "Not Found!";
}
}
$string = "BitDegree makes learning fun";
$search = "fun";
echo look($search, $string);
?>
Note: When using PHP strpos(), keep in mind the first position in a string is not 1, but 0.
str_replace()
This function is used to have a part of PHP string replaced. When you add str_replace
to a PHP string, the method searches for specified text inside it. If found, it is changed to the text you indicated:
<?php
echo str_replace("John", "Ben", "My name is John Johnson");
// The first value we input, i.e. "John" is the text we want to replace
// The second value we input, i.e. "Ben" is the text we want to use to replace the first text
// The third value is the string we want to use the function on
?>
PHP String: Summary
- A character sequence surrounded by quotes is called a string. It is one of the most basic data types.
- The number of characters one string consists of is called PHP string length. It can be fetched using
strlen()
function. - PHP offers more inbuilt functions for processing strings. To learn more methods than this tutorial presented, you should read our other tutorials on string manipulation in PHP.