Living in the age of media, we are surrounded by massive amounts of information. PHP RSS parser allows people to receive updated or new data from different websites quickly. If you don't want to miss news articles of a specific kind, you can use RSS to receive updated content automatically.
RSS stands for Really Simple Syndication. It collects the headlines from news systems, turns their format into XML and displays the complete feed in your computer. Unlike a newsletter, RSS don't require you to provide your email address, so you not only save time but also keep your privacy.
Contents
PHP RSS Parser: Main Tips
- You can build an interactive PHP RSS feed reader web application (also called a parser) using PHP, AJAX and RSS.
- PHP RSS readers are meant for reading PHP RSS feeds that contain frequently updated info (such as news).
- PHP RSS feeds are published not only by the big news websites: a lot of bloggers do it too. Some content systems (such as Wordpress) provide this opportunity by default.
- PHP RSS feed are shown in HTML or XML strings. A PHP RSS parser translates it into a DOM document.
Creation Process Explained
We're sure you got the idea what this PHP RSS parser is. To teach you how it is created, we have to cover some basic instructions. Let's go through the files you need to learn.
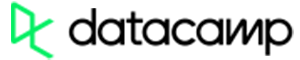
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
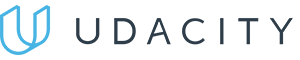
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
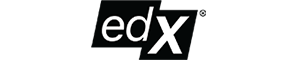
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
HTML File
First, a user has to select a particular RSS feed from a dropdown. To do that, we call a function called show_rss()
. This function can be triggered by the onchange
event:
<html>
<head>
<script>
function show_rss(str) {
if (str.length == 0) {
document.getElementById("rss_output").innerHTML = '';
return;
}
if (window.XMLHttpRequest) {
//if the field is not empty, create an XMLHttpRequest to get suggestions
xmlhttpreq = new XMLHttpRequest();
} else {
// server response is ready, we call the function
xmlhttpreq = new ActiveXObject("Microsoft.XMLHTTP");
}
xmlhttpreq.onreadystatechange = function() {
if (this.readyState==4 && this.status==200) {
document.getElementById('rss_output').innerHTML = this.responseText;
}
}
xmlhttpreq.open('GET', 'get_rss.php?q=' + str, true);
xmlhttpreq.send();
}
</script>
</head>
<body>
<form>
<select onchange="show_rss(this.value)">
<option value="">Select the RSS feed title:</option>
<option value="google_news">Google News</option>
<option value="bbc_news">BBC News</option>
</select>
</form>
<br>
<div id="rss_output">This is where the RSS feed data goes.</div>
</body>
</html>
Let's break down all the steps that the show_rss()
function takes for the PHP RSS feed reader to work:
- First, it checks whether a selection has been made.
- An XMLHttpRequest object is created.
- A function is then set up. It must be ready to execute once the server response is prepared.
- Finally, the request is sent to the PHP document get_rss.php located on our server.
Note: pay attention when you apply q parameter to the get_rss.php?q="+str.
PHP File
JavaScript accesses get_rss.php by making a call to the server, which then does this:
<?php
//get q parameter from the URL
$q = $_GET["q"];
//check which feed was selected
if($q == 'reuters_news') {
$xml_src=("http://news.google.com/news?ned=us&topic=h&output=rss");
} elseif($q == 'bbc_news') {
$xml_src=("http://feeds.bbci.co.uk/news/world/rss.xml");
}
$xml_doc = new DOMDocument();
$xml_doc->load($xml_src);
//get elements from inside the "<channel>"
$c=$xml_doc->getElementsByTagName('channel')->item(0);
$c_title = $c->getElementsByTagName('title')
->item(0)->childNodes->item(0)->nodeValue;
$c_link = $c->getElementsByTagName('link')
->item(0)->childNodes->item(0)->nodeValue;
$c_desc = $c->getElementsByTagName('description')
->item(0)->childNodes->item(0)->nodeValue;
//output elements from "<channel>"
echo("<p><a href='" . $channel_link
. "'>" . $channel_title . "</a>");
echo("<br>");
echo($channel_desc . "</p>");
//get and output "<item>" elements
$x = $xmlDoc->getElementsByTagName('item');
for ($i=0; $i< = 2; $i++) {
$item_title = $x->item($i)->getElementsByTagName('title')
->item(0)->childNodes->item(0)->nodeValue;
$item_link = $x->item($i)->getElementsByTagName('link')
->item(0)->childNodes->item(0)->nodeValue;
$item_desc = $x->item($i)->getElementsByTagName('description')
->item(0)->childNodes->item(0)->nodeValue;
echo ("<p><a href='" . $item_link
. "'>" . $it_title . "</a>");
echo ("<br>");
echo ($item_desc . "</p>");
}
?>
When Javascript requests PHP RSS feeds, this is what goes down:
- The system checks which RSS feed has been selected.
- New XML DOM object is created.
- PHP RSS Parser loads the RSS file inside the XML variable we have.
- Next, it parses the elements from the channel element.
- Elements from the item elements are extracted and displayed.
PHP RSS Parser: Summary
- With a bit of practice using PHP, AJAX and RSS, you can create a custom application for reading RSS feeds.
- RSS feeds usually contain information that gets updated often. They are usually published by news websites and blogs.
- RSS feeds are displayed in HTML or XML strings, which the reader can then parse into a DOM document.