We have already learned about XML format. Therefore, understanding JSON will be much easier: XML and JSON are similar. They are both file formats designed to contain plain text and be easily read by both machines and humans. Therefore, they are used as standards.
If you need to retrieve specific information from the server and make it appear on your website, you will probably be using JSON. The name of JSON stands for JavaScript Object Notation. It is based on JavaScript, so having some basic knowledge of it would be greatly welcome here. JSON also uses JavaScript syntax.
Unlike XML, it does not support nametags and comments, but does support PHP arrays. JSON is also more lightweight and easier to read. In this tutorial, we will view a lot of examples and learn to use the most basic PHP JSON functions called PHP json_decode() and json_encode().
Contents
PHP json_decode() and json_encode(): Main Tips
- JSON is anonymous data that can be translated in PHP variables.
- Arrays can be converted to JSON format.
- JSON is commonly used for reading data out of a web server and displaying it on a website.
- There are integrated functions to manipulate JSON. Most important of them are PHP
json_encode()
and PHPjson_decode()
.
Learn to Encode Files
To convert PHP objects into JSON, we use PHP json_encode()
function. To do an opposite conversion, PHP json_decode()
is used, but more on that later. Now let's see a code example:
<?php
$myObj->fruit = "Banana";
$myObj->expires = 2017;
$myObj->country = "Lithuania";
$exampleJSON = json_encode($myObj);
echo $exampleJSON;
?>
The Client JavaScript
This JavaScript uses AJAX to get the PHP file:
var xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function(){
if (this.readyState == 4 && this.status == 200) {
var newObj = JSON.parse(this.responseText);
document.getElementById("JSON").innerHTML = newObj.fruit;
}};
xmlhttp.open("GET", "demo.php", true);
xmlhttp.send();
How Arrays Are Converted
Just like objects, arrays are translated into JSON using PHP json_encode()
function.
<?php
$myArr = array("Bob", "Bill", "Ben", "Brutus");
$myJSON = json_encode($myArr);
echo $myJSON;
?>
The Client JavaScript
This JavaScript uses AJAX to get the PHP file out of the array:
var xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
var newObj = JSON.parse(this.responseText);
document.getElementById("JSON").innerHTML = newObj[2];
}
};
xmlhttp.open("GET", "demo_array.php", true);
xmlhttp.send();
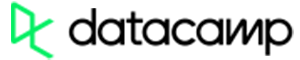
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
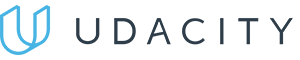
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
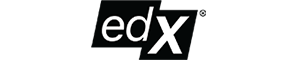
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Making Requests to Database
As a coding language, PHP JSON is server-side. It means that you can only use it for tasks that the server executes. Connecting to a database could be one of those appropriate tasks. Imagine we have a database that holds certain information. To ask for it, we need to call the database:
<!DOCTYPE html>
<html>
<body>
<h2>JSON data from PHP file.</h2>
<p>The JSON received from the PHP file:</p>
<p id="example"></p>
<script>
var obj, dbParameters, xmlhttp;
obj = { "table":"fruits", "limit":5 };
dbParam = JSON.stringify(obj);
xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("example").innerHTML = this.responseText;
}
};
xmlhttp.open("GET", "your_file.php?x=" + dbParam, true);
xmlhttp.send();
</script>
</body>
</html>
Example explained:
- First, we describe the object holding the properties table and limit, and translate it to JSON.
- After that, a call holding a JSON parameter is sent to a PHP file.
- When the call is returned, we output what we received from the file.
Here we have our PHP file:
<?php
header("Content-Type: application/json; charset=UTF-8");
$obj = json_decode($_GET["x"], false);
$connect = new mysqli("RequiredServer", "RequiredUsername", "RequiredPassword", "Northwind");
$result = $connect->query("SELECT name FROM ".$obj->table." LIMIT ".$obj->limit);
$output = array();
$output = $result->fetch_all(MYSQLI_ASSOC);
echo json_encode($output);
?>
Let's review the script above step by step:
- The call is translated to an object using PHP
json_decode()
. - A connection to a database is established in order to write data to an array.
- Array is attached to an object, which is returned in JSON format using PHP
json_encode()
.
Looping Through the Results
The data in the file containing the PHP code can be converted into a JavaScript array. To do that, we will be using PHP for loop:
...
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
newObj = JSON.parse(this.responseText);
for (x in newObj) {
txt += newObj[x].name + "<br>";
}
document.getElementById("JSON").innerHTML = txt;
}
};
...
Usage of POST Method
Whenever you need to send certain information to the server, HTTP POST
method seems like the best possible choice. To use it for an AJAX call, don't forget to define the method and the right header. The data that we need to be sent to the server will become the argument for the send()
function:
obj = { "table":"customers", "limit":10 };
dbParam = JSON.stringify(obj);
xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
newObj = JSON.parse(this.responseText);
for (x in newObj) {
txt += newObj[x].name + "<br>";
}
document.getElementById("JSON").innerHTML = txt;
}
};
xmlhttp.open("POST", "JSON.php", true);
xmlhttp.setRequestHeader("Content-type", "application/learn-encoded");
xmlhttp.send("x=" + dbParam);
When working with PHP files, only the method differs:
<?php
header("Content-Type: application/json; charset=UTF-8");
$obj = json_decode($_POST["x"], false);
$conn = new mysqli("myServer", "myUser", "myPassword", "Northwind");
$result = $conn->query("SELECT name FROM ".$obj->table." LIMIT ".$obj->limit);
$outp = array();
$outp = $result->fetch_all(MYSQLI_ASSOC);
echo json_encode($outp);
?>
PHP json_decode() and json_encode(): Summary
- JSON is a standard text-based format, similar but more lightweight than XML. It holds anonymous data that may be parsed into variables.
- It is often used to read server data and output it in a webpage.
- JSON can be handled by using inbuilt PHP functions. For example, a PHP object might be turned into JSON format file using PHP
json_encode()
function. For the opposite transformation, use PHPjson_decode()
. - PHP arrays are not supported by XML but can be converted into JSON.