If you fail to apply PHP filtering (sanitizing and validating) to your data correctly, security issues may arise. It is especially important to consider external data which doesn't always come from secure sources.
All the data coming from outside the code should always be validated and sanitized to prevent data insertion and other user-input related security risks. External data also comes from servers and databases.
PHP filtering sanitizes data by getting rid of all illegal characters. Also, it can validate data, checking whether the information is in the right form.
Contents
PHP Filtering: Main Tips
- PHP includes inbuilt filter functionality, allowing to validate or sanitize data you enter through forms or otherwise.
- This is extremely useful if you want to use special characters as text without triggering code, preventing data insertion.
- Validating data means determining whether the data is in correct form.
- Sanitizing data means to remove of any special characters from the data.
Using filter_lists()
To see what the functionality of PHP filters offers, use the filter_lists()
function as you can see in the code example below:
<ul>
<?php
foreach (filter_list() as $id => $filter) {
echo '<li>' . $filter . ', ' . filter_id($filter) . '</li>';
}
?>
</ul>
filter_var() Function Explained
A function that validates and sanitizes data is called filter_var()
. It takes two variables upon being initialized. First parameter indicates which variable to sanitize. The second specifies the filter that is to be used.
Reasons to Sanitize Data
There are many uses for external input in web applications:
- User-defined input from forms.
- Cookie data.
- Web service data.
- Server variable data.
- Results from database queries.
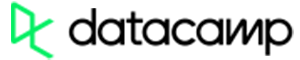
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
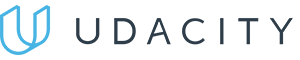
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
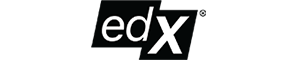
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Making Filter Input
Using code examples, we will now explain how PHP filtering can be used on various types of external data. Let's go over them step by step.
Strings and Integers
The example below shows how filter_var()
is used to PHP filter sanitize strings:
<?php
$str = "<h1>Hey!</h1>";
$newstring = filter_var($str, FILTER_SANITIZE_STRING);
echo $newstring;
?>
The code example below illustrates how filter_var()
is used to check if a variable is an integer, along with displaying messages according to the outcome:
<?php
$int = 87;
if (!filter_var($int, FILTER_VALIDATE_INT) === false) {
echo "This is an integer.";
} else {
echo "This is not an integer. Or at least not a valid one. Read more about that.";
}
?>
A slight issue might arise here though. If the value equals 0 (even when the variable is an integer), filter_var()
will return false
. Use this code to work around it:
<?php
$int = 0;
if (filter_var($int, FILTER_VALIDATE_INT) === 0 || !filter_var($int, FILTER_VALIDATE_INT) === false) {
echo "This is an integer.";
} else {
echo "This is DEFINiTELY not an integer";
}
?>
IP Addresses, Emails and URLs
Carefully review the code snippet provided. In it, we use filter_var()
to check if a variable is a valid IP address. You can see both possible responses in the code. Which one will be displayed, depends on the results of validation:
<?php
$ip = "127.0.0.1";
if (!filter_var($ip, FILTER_VALIDATE_IP) === false) {
echo "Pretty sure that $ip indeed is working, valid IP address.";
} else {
echo "$ip ? Nope. NOT a valid IP address, I can tell you that much.";
}
?>
In the example below, you can see we use PHP filter_var()
to make PHP sanitize form data (remove any illegal symbols from the e-mail field) and validate whether it is a properly formatted e-mail address:
<?php
$email = "[email protected]";
// Remove all illegal characters from email
$email = filter_var($email, FILTER_SANITIZE_EMAIL);
// Validate e-mail
if (!filter_var($email, FILTER_VALIDATE_EMAIL) === false) {
echo "$email is working, valid e-mail address you can send letters to.";
} else {
echo "$email is DEFINITELY not an e-mail address";
}
?>
Now, in the next example, PHP filter_var()
is again used for both validation and sanitizing, but this time the object is a URL address. Take a look:
<?php
$url = "https://www.bitdegree.org/learn/";
// Remove all illegal characters from a url
$url = filter_var($url, FILTER_SANITIZE_URL);
// Validate url
if (!filter_var($url, FILTER_VALIDATE_URL) === false) {
echo "$url is TOTALLY legit. Really, it's a valid URL.";
} else {
echo "$url is DEFINITLELY not a valid, working URL";
}
?>
PHP Filtering: Summary
- Inbuilt PHP filtering functionalities lets you easily validate and sanitize the data that either you enter or users input.
- Validating means finding our whether the data in question is in the right format.
- Sanitizing gets rid of all the special characters the data might contain. By making PHP sanitize form data, you make sure you're only getting the input the intended to.