PHP errors don't reach the user-side that often, but developers encounter them daily. When you are programming and see PHP show errors, your projects get delayed as you are forced to fix specific issues.
Even newbies are familiar with the most basic errors: a specific page not found, a URL handle typed incorrectly, etc. The browser shows an error message that contains a filename, line number, and a note describing the detected error. There is no need to fear PHP errors since there are methods to help you manage them.
Contents
PHP Show Errors: Main Tips
- Error handling is an integral part of coding any web application that has user input.
- A basic PHP error handler example would be a simple conditional statement that assigns a PHP error message to an empty string placed next to the input field, specifying what the error was.
What Errors Are and How to Handle Them
While you learn what a PHP error is, you need to know that they come in two types: internal and external. Internal errors are the ones developers accidentally leave in their scripts. To avoid them, you need to be careful. External PHP errors are the ones that arise outside the code (for example, failing to connect to a database).
There are three basic methods when it comes to PHP error handling:
- A basic
die()
statement. - Defining your own error messages and alerts (making PHP show errors).
- Reporting errors.
Using die() Function
The example below displays a script opening a text file:
<?php
$doc = fopen('helloworld.txt', 'r');
?>
In case the file mentioned doesn't exist, this is the message you will be shown:
Warning: fopen(helloworld.txt) [function.fopen]: failed to open stream:
No such file or directory in C:\dir\error_handling.php on line 2
To prevent the user from being shown that default error message, we should check whether the file exists before trying to access it:
<?php
if(!file_exists('helloworld.txt')) {
die('No such file exists!');
} else {
$doc = fopen('helloworld.txt', 'r');
}
?>
After making modifications to the script, this is the error message that will be displayed:
File not found
This method is much more efficient than letting the script run into a PHP error. The function stops the code from performing a command by checking for its prerequisite (whether the needed file exists at all). If the action cannot be completed, the script is stopped. Also, a custom error message is set to be displayed. Merely stopping the script is not recommended though.
Checkers and Handlers
If you doubt your own ability to see internal errors in the code, you may use various PHP error checkers. You can find them online (for example, PHP Code Checker) or download a specific software (such as Phan). A fresh pair of eyes is always useful, and a PHP error checker will make PHP show errors you did not notice.
An error handler is a custom function, which is called whenever a PHP error occurs. It is not challenging to create. You must note that a custom error handler must be able to handle at least two parameters passed into it (error message and level are required). The number of parameters might reach up to five, as there are three optional ones (file, line-number, and context) that might be included.
Let's look at the syntax example:
err_function(err_level,err_message, err_file,err_line,err_context)
<?php
$text1 = "Learning PHP";
$text2 = "ALL the PHP";
$var1 = 58;
$var2 = 4;
print "<h6>" . $text1 . $text2 . "</h6>";
print $var1 + $var2;
?>
The following table consist of the parameters you can add:
Parameter | Description |
---|---|
error_level | Required. A numeric value which is used to tell the level of the report. |
error_message | Required. A string value which is outputted upon an error occurring. |
error_file | Optional. The name of the file in which the error took place. |
error_line | Optional. The number of the line on which the error took place. |
error_context | Optional. Used to specify using which variables, files, functions the error occurred in. |
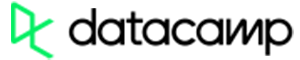
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
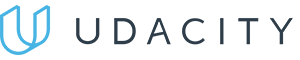
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
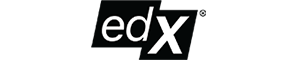
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Report Levels
View the table below. It will explain different PHP error levels that an error report might list:
Value | Constant | Description |
---|---|---|
2 | E_WARNING | Minor, non-fatal error, which occurs while running the script and does not stop it. |
8 | E_NOTICE | This includes run-time notices, which could include minor errors and notices you may get when normally running the script. |
256 | E_USER_ERROR | Fatal error generated by the user. Much like E_ERROR, set by the author of the script by calling the trigger_error() function. |
512 | E_USER_WARNING | Non-fatal warning generated by the user. Much like E_WARNING, set by the author of the script by calling the trigger_error() function. |
1024 | E_USER_NOTICE | Notice generated by the user. Much like E_NOTICE, set by the author of the script by calling the trigger_error() function. |
4096 | E_RECOVERABLE_ERROR | Fatal error that can be caught. Much like E_ERROR, however, ir can be caught by a user-defined error handler, which can be set up using the set_error_handler() function. |
8191 | E_ALL | Every error and warning (E_STRICT became a part of E_ALL in PHP version 5.4) |
Let's try creating a basic PHP error handling function:
function custom_error($err_no, $err_str) {
echo "<b>Error caught!</b> [$err_no] $err_str<br>";
echo "Script end!";
die();
}
Upon triggering, this function gets the error level and message, which it then outputs and terminates the code.
Now that we have a custom way of handling PHP errors, we need to specify when it should be used.
Setting up a Handler
There is a default, built-in error handler in PHP. However, we are going to make our custom-made error handler the default one.
We should keep in mind that an error handler may be specialized for certain errors, allowing us to handle specific errors. In this case, our handler will make PHP show all detected errors:
set_error_handler("custom_error");
Given that this error handler is designed for all errors, set_error_handler()
only needs to hold a single parameter: the purpose of the second parameter is to specify the level of errors this particular handler would apply to, and ours is universal (therefore, no need for specification).
We can test the error handler by passing a variable that does not exist in current context.
<?php
//error handler function
function custom_error($err_no, $err_str) {
echo "<b>Error caught!</b> [$err_no] $err_str";
}
//set error handler
set_error_handler("custom_error");
//trigger error
echo($test);
?>
The desired output is making PHP show errors that occured:
Error: [8] Undefined variable: test
Triggering an Error
When dealing with user input, it is often handy to trigger a PHP error whenever illegal input is used. You can do this by using the trigger_error()
function.
Look at the example below. The script will check the $test
variable. If it meets the required criteria (is bigger than 1), the script makes PHP show errors:
<?php
$test = 3;
if ($test >= 1) {
trigger_error('The value has to be 1 or lower');
}
?>
Here is the output we should be getting:
Notice: Value has to be 1 or lower in C:\dir\error_handling.php on line 6
Following these steps, you can trigger a PHP error anywhere you like in your script. The second parameter will allow you to set the level of the error that occurs.
Now, in this example, E_USER_WARNING
is set to occur if the $test
happens to meet the same criteria as in the previous example. When E_USER_WARNING
is generated, we will use a user-defined error handler to terminate the script:
<?php
//error handler function
function custom_error($err_no, $err_str) {
echo "<b>Error caught!</b> [$err_no] $err_str<br>";
echo "Script end";
die();
}
//set error handler
set_error_handler('custom_error', E_USER_WARNING);
//trigger error
$test = 3;
if ($test >= 1) {
trigger_error('Value has to be 1 or lower', E_USER_WARNING);
}
?>
This is the output we should be getting:
Error caught! [512] Value has to be 1 or lower
Script end
Logging Errors
We have gone over creating error handlers and triggering PHP errors, so making PHP show all errors is now rather clear. Let's take a closer look at the way they are logged.
By default, in PHP an error log is sent into the logging system of the server or a document, which depends on the configuration of the error log inside your php.ini file. By using a function called PHP error_log()
, you can modify where the error logs are sent.
A lot of developers recommend sending error logs to yourself via e-mail. In this way, you will be able to see what is happening on your website whenever you check your e-mail. In the example below, we set up a PHP error handler to send us an e-mail whenever an error is caught:
<?php
//error handler function
function custom_error($err_no, $err_str) {
echo "<b>Error caught!</b> [$err_no] $err_str<br>";
echo "Site owner notified";
error_log("Error caught! [$err_no] $err_str", 1, "[email protected]", "From: [email protected]");
}
//set error handler
set_error_handler('custom_error', E_USER_WARNING);
//trigger error
$test = 2;
if ($test >= 1) {
trigger_error('Value has to be 1 or lower', E_USER_WARNING);
}
?>
Here is the output we should be getting:
Error caught! [512] Value has to be 1 or lower
Site owner notified
The message could look like this:
PHP Error Handling Example #14
However, minor errors occur regularly, so it might be more practical to let the default logging system log them.
PHP Show Errors: Summary
- Any application that allows user input requires the developer to know how to handle errors.
- It's handy to trigger an error in case of illegal input.
- A simple conditional statement that assigns a message, specifying the detected error, can be considered a basic error handler. The message is displayed in an empty string placed next to the input field.
- Using PHP
error_log()
function, you can modify the destination of error logs.