Contents
PHP Date Format: Main Tips
- This date() function is used in formatting date and time in your code.
- There are special formatting characters: PHP year, week, day, etc.
- You can use it to make PHP get timestamp automatically and update the copyright dates on your website.
Syntax Rules Explained
The syntax rules for entering a date are rather simple:
date(format, timestamp)
The timestamp
part describes an integer Unix timestamp. Entering it is optional - if you don't do it, it will result in making PHP get timestamp automatically and display the current local time (time()
).
Now, for the format
part, it's a different story. It is necessary to enter because that's how you specify how your PHP date formats will look.
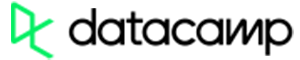
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
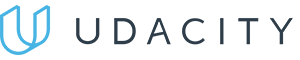
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
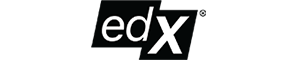
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Common Formatting Characters
In PHP years, months and days are often referred to by using special symbols. There are four characters most commonly used with PHP date function:
- d (01-31) represents the day number.
- m (01-12) represents a month number.
- Y (four digits) represents a year number.
- l (lowercase 'L') indicates the day of the week.
More symbols (/
, .
, -
) can be added for additional PHP date formatting.
In the code example below, you can see four different ways to create PHP date formats:
<?php
echo "Today is " . date("Y/m/d") . "<br>";
echo "Today is " . date("Y.m.d") . "<br>";
echo "Today is " . date("Y-m-d") . "<br>";
echo "Today is " . date("l");
?>
Now, the four characters listed above might be the most commonly used with datetime PHP function, but your choices are not limited to them. Let's have a look at the whole list of characters you can use for PHP date formats and what they indicate:
Character | Description |
---|---|
d | The day of the month (01-31) |
D | Shows a day in three letter text |
j | Day of the month (without zeros in front; 1-31) |
l (lowercase 'L') | Displays the full name of a day |
N | Week day displayed in a numeric format (1 for Monday, 7 for Sunday) |
S | The suffix for a day of the month. (st, rd, th or nd) Can be combined with j to create 1st; 13th, etc. |
w | Week day displayed in a numeric format (0 for Sunday, 6 for Saturday) |
z | Number for a day of the year (0-365) |
W | The number for the current week of year (weeks start on Monday) |
F | A full name of a month (January, December, etc.) |
m | The number of a current month (01-12) |
M | The name of a month written in three letters |
A numeric representation of a month, without leading zeros (1-12) | |
t | Displays a number of days in the current month |
L | Displays 1 if it is a leap year, and 0 if it is not. |
o | The ISO-8601 year number |
Y | The current year displayed in four digits |
y | The current year displayed in two digits (the last two) |
a | Displays the time prefix (am/pm) |
A | Displays the time prefix in uppercase (AM/PM) |
B | Internet swatch time (000-999) |
g | Hours displayed in 12-hour format (1-12) |
G | Hours displayed in 24-hour format (0-23) |
h | Hours displayed in 12-hour format with leading zero (01-12) |
H | Hours displayed in 24-hour format. Uses leading zeros (00-23) |
i | Minutes displayed with leading zeros (00-59) |
s | Seconds displayed with leading zeros (00-59) |
u | Displays microseconds |
e | Displays the timezone (UTC, GMT, etc.) |
I (capital i) | Displays 1 if the date is in daylight savings time and 0 if it is not |
O | Displays the difference from the Greenwich time (GMT) (e.g.: +0200) |
P | Displays the difference from the Greenwich time (GMT) in hours:minutes |
T | Timezone abbreviations (e.g.: MDT, EST) |
Z | The timezone offset in seconds. From -43200 to 50400 (to the west from UTC is negative) |
c | The date in ISO-8601 format (e.g.: 2017-09-12T19:54:40+00:00) |
r | The date in RFC 2822 format (e.g.: Fri, 13 Jan 2017 18:09:10 +0200) |
U | Displays how many seconds had passed since Unix Time (January 1st, 1970, 00:00:00 GMT) |
List of Predefined Constants
There are also a few predefined constants for datetime PHP that developers use. Take a look at the table below:
Constant | Description |
---|---|
DATE_ATOM | Atom (e.g.: 2017-09-12T19:12:11+00:00) |
DATE_COOKIE | HTTP Cookies (e.g.: Monday, 11-Apr-11 19:58:51 UTC) |
DATE_ISO8601 | ISO-8601 (example: 2017-09-12T12:12:11+0000) |
DATE_RFC822 | RFC 822 (e.g.: Mon, 11 Apr 14 19:11:15 +0000) |
DATE_RFC850 | RFC 850 (e.g.: Tuesday, 11-Apr-10 11:12:51 UTC) |
DATE_RFC1036 | RFC 1036 (e.g.: Mon, 11 Apr 11 19:42:31 +0000) |
DATE_RFC1123 | RFC 1123 (e.g.: Mon, 10 Apr 2018 13:32:01 +0000) |
DATE_RFC2822 | RFC 2822 (e.g.: Fri, 10 Apr 2017 18:53:31 +0000) |
DATE_RFC3339 | Same as DATE_ATOM (since PHP 5.1.3) |
DATE_RSS | RSS (e.g.: Mon, 12 Aug 2017 13:22:21 +0000) |
DATE_W3C | World Wide Web Consortium (e.g.: 2017-09-12T13:32:11+00:00) |