Even though it might sound very similar, knowing how to open PHP files is not the same as knowing how to open a file using PHP. In the first case, PHP code is an object you open, and in the latter, it acts as a tool for opening external files.
Knowing how to open a PHP file is easy: it is a text document in its essence, so you can open it with any text editor or web browser. Notepad is okay, a dedicated PHP editor is even better. A web browser also works. Now, opening external files using PHP is a bit different but not any less important.
Opening and closing files is crucial for web applications to work correctly. PHP has a set of useful functions for that. You are already familiar with the PHP fopen(). Now, we will introduce a few more and highlight their differences.
Contents
PHP fopen: Main Tips
- When opening files, PHP
fopen()
function is preffered fromreadfile()
by most as it gives the coder more options. It it also commonly used by developers who coded in C or C++ languages before since the tools in those are more or less the same. - If you are having problems with file opening through PHP, it is advised to check whether file access is granted to PHP.
fopen()
andfclose()
functions are used for opening and closing files.fread()
,fgets()
,feof()
andfgetc()
functions are used to PHP read file information.
Functions to Apply
For the PHP file manipulation, you have an array of potential functions. You should start exploring these methods and finding out in which situations it is best to apply specific functions. For instance, there are separate methods for opening, reading and analyzing files.
fopen()
PHP fopen()
function is used to create or open a file in a selected mode.
<?php
$demofile = fopen('text_file.txt', 'r') or die('File cannot be opened!');
echo fread($demofile, filesize('text_file.txt'));
fclose($demofile);
?>
fread()
This function lets you PHP read file, but only shows a selected maximum number of bytes in an opened file.
<?php
fread($demofile, filesize('text_file.txt'));
?>
fgets()
This function also lets you PHP read file, but fetches a single line from a file. Often used to make PHP read file line by line.
<?php
$demofile = fopen('text_file.txt', 'r') or die('File cannot be opened!');
echo fgets($demofile);
fclose($demofile);
?>
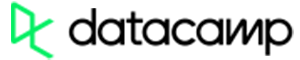
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
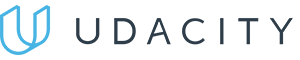
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
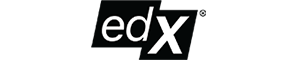
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
fgetc()
fgetc()
function is also meant to PHP read files. The difference is that it is used to only read a single character from a file.
<?php
$demofile = fopen('text_file.txt', 'r') or die('File cannot be opened!');
// Output characters one by one
while(!feof($demofile)) {
echo fgetc($demofile);
}
fclose($demofile);
?>
Note: After a call to the fgetc() function, the file pointer moves to the next character.
fclose()
PHP files are closed using fclose()
function.
<?php
$demofile = fopen('text_file.txt', 'r');
// Code....
fclose($demofile);
?>
feof()
This function is meant to check if the end of file has been reached.
<?php
$demofile = fopen('text_file.txt', 'r') or die('File cannot be opened!');
// Output file lines one by one
while(!feof($demofile)) {
echo fgets($demofile) . "<br>";
}
fclose($demofile);
?>
Note: The feof() function proves itself handy in situations when the length of the file is unknown.
Modes to Open Files
When you use fopen PHP methods, you can open files in different modes. They can either be accessible for reading or ready to be modified. There are eight modes in total: r, r+, w, w+, a, a+, x, and x+. Let's break them down:
- r mode opens a file in read-only mode. The cursor is placed in the beginning of it by default. r+ acts almost the same, but you can both read the file and write in it.
- w mode opens a file as write-only. The cursor is again placed in the beginning automatically. w+ performs the same, but also allows to PHP read the file.
- a opens a file in write-only mode as well, but the cursor is now placed at the end. a+ acts almost the same, but you can both read the file and write in it. If the file is not found, both modes create a new one.
- x is used for creating new files. They appear having a write-only mode (choose x+, if you wish to also be able to read it). If a file with such name is found, the function fails and returns False.
Tip: If you haven't noticed it yourself, a plus symbol (+) clearly indicates that a file is suitable for both reading and writing.
And that's about it! If it is still a bit unclear to you how to open a PHP file that contains the code itself, we'd recommend reading a lesson on how to install PHP: it has useful tips for the beginners and recommends some systems for a smooth start.
PHP fopen: Summary
- Using
fopen
PHP function gives you more functionality thanreadfile()
. It's also similar to functions used in C or C++ languages. - Always make sure PHP is granted file access.
- Use
fgets()
whenever you need to make PHP read file line by line. fread()
,fgets()
,feof()
andfgetc()
functions are meant to make your code read the information of the file.