TL;DR — Responsive images refer to images that properly adjust to different screen sizes, resolutions, positions, and other factors. Since the majority of websites are responsive, you should avoid adding static images to pages.
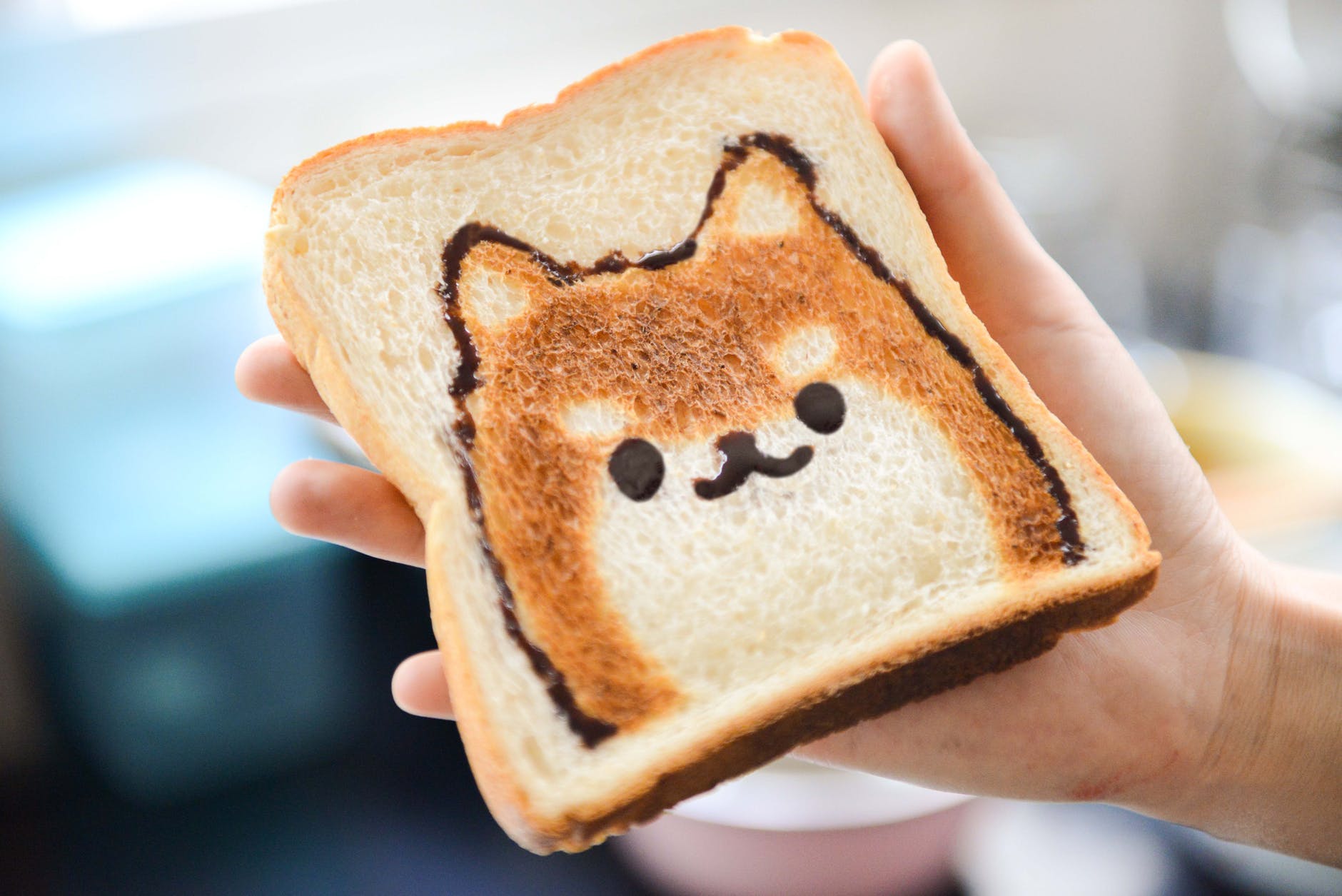
Contents
- 1. Adding responsive images with CSS
- 2. Specifying the width for scaling
- 3. Setting background-size to fit screen
- 4. More on the background image
- 4.1. Keeping the aspect ratio
- 4.2. Stretching images to fill the area
- 4.3. Covering the area, but keeping the aspect ratio
- 5. Responsive images and media queries
- 6. HTML5 <picture> element
- 7. Responsive image: useful tip
Adding responsive images with CSS
Responsive web design resizes or changes the orientation of the window without losing content quality. Images scale responsively after you set certain CSS width properties.
There are three main methods for styling CSS background images. You can use different image files based on the viewport size of devices.
Specifying the width for scaling
CSS images sizes change in response to different dimensions of the browser window. You need to set either width or max-width
properties for CSS to respond to such changes.
You can enable scaling down and up by setting the width
to 100%.
In the example above, the image can be scaled up until it becomes larger than the original size. Therefore, images might have poorer quality on bigger screens.
To prevent responsive images from becoming bigger than their original CSS image sizes, use max-width
, and set it to 100%.
Setting background-size to fit screen
Using CSS, you can set the background-size
property for the image to fit the screen (viewport).
The background-size
property has a value of cover
. It instructs browsers to automatically scale the width
and height
of a responsive background image to be the same or bigger than the viewport.
In this code example, we make the CSS background image size fit the screen:
html {Â Â Â
background: url('image.png') no-repeat center fixed; Â Â Â
background-size: cover;
}
- The responsive background image fills the whole page without leaving whitespace.
- The image is at the center, scales if necessary, and does not require a scroll bar.
More on the background image
Keeping the aspect ratio
Choose this method if you have a small image and want to keep its quality.
Set the background-size
property to contain. It tells the browser that the background image scales trying to fit the content area, but does not lose its aspect ratio or get blurry.
div {
width: 100%;
height: 300px;
background-image: url('doggo.jpg');
background-repeat: no-repeat;
background-size: contain;
border: 2px solid #e9385a;
}
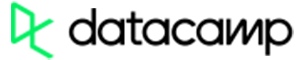
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
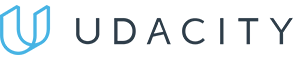
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
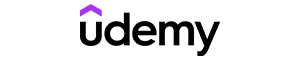
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Stretching images to fill the area
You can set the background-size
property to 100% 100% to make the image stretch to fit a specific area:
div {
width: 100%;
height: 300px;
background-image: url('doggo.jpg');
background-size: 100% 100%;
border: 2px solid #e9385a;
}
Covering the area, but keeping the aspect ratio
To cover the area with the background image and keep its aspect ratio, you can set the background-size
property to cover. It may cut off a part of the image to keep it proportional.
div {
width: 100%;
height: 300px;
background-image: url('doggo.jpg');
background-size: cover;
border: 2px solid #e3985a;
}
Responsive images and media queries
Large images on small screens can make the user-experience worse by loading the page longer.
For this reason, we use media queries to display images differently on various screen-sizes.
There are two images (one larger and one smaller) in the following example. CSS chooses to display the one appropriate fitting the screen size:
/* For screens with width smaller than 400px */
body {
background-image: url('small-birb.jpg');
}
/* For larger screens */
@media only screen and (min-width: 400px) {
body {
background-image: url('birb.jpg');
}
}
Instead of min-width
, you can use media query called min-device-width
. It works responsively with the device viewport width.
Therefore, it makes sure that the image does not change when you resize the browser window:
/* For devices with width smaller than 400px */
body {
background-image: url('birb-small.jpg');
}
/* For larger devices */
@media only screen and (min-device-width: 400px) {
body {
background-image: url('birb.jpg');
}
}
HTML5 <picture> element
The <picture>
element was introduced in HTML5. It is for defining two or more images.
Remember: the <picture> provides options for showing different images when one image cannot load or when image size depends on the screen size.
<picture>
<source srcset="birb-small.jpg" media="(max-width: 400px)">
<source srcset="doggo.jpg">
<img src="doggo.jpg" alt="cannot display">
</picture>
You must set the srcset
attribute to specify the image source. You can set as many sources as you need, but have in mind that the order in which you specify them matters. The first source to fit the preferences of your code is used.
The media
attribute is not required, but you should set it to accept the media queries. You should also define the <img>
element for older browsers that don't support HTML5 and <picture>
element.
Responsive image: useful tip
- You can also make responsive images using Bootstrap. It makes images adjust to screen sizes by adding
.img-fluid
class, not theimg-responsive
(it is from the Bootstrap 3).