TL;DR – depending on the method you choose, you can either sort an existing list in Python, or create a sorted one without changing the original.
Contents
How to sort a list in Python
The easiest way to make Python sort lists is using the sort
function. It will modify the original list without creating a new one:
The system will sort numbers in an ascending order and strings in an alphabetical order. You can reverse this by including an optional argument reverse=True
:
pies = ['Pecan', 'Apple', 'Cherry', 'Keylime']
pies.sort(reverse=True)
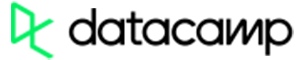
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
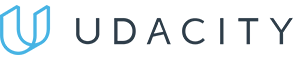
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
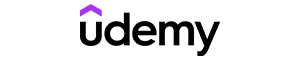
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Creating a Python sorted list
In some cases, you might want to leave the original list untouched, but still need a sorted version of it. To achieve this, use the sorted
method:
pies = ['Pecan', 'Apple', 'Cherry', 'Keylime']
sorted_list = sorted(pies)
Just like with sort
, you can use the reverse
argument to make Python sort list in reverse order. You can also sort it according to a different criteria by using an optional key
argument and defining a basis for sorting.
In the example below, we create a Python sorted list according to the length of the string:
pies = ['Blackberries', 'Pecan', 'Lime', 'Cinammon']
sorted_list = sorted(pies, key=len)
Python sort list: useful tips
- Sorting a list in alphabetical order can be performed wrong when you're dealing with both uppercase and lowercase letters, and the system treats them as if they were different in value. To work around this, use the key argument and define
str.lower
as the basis for sorting. - When working with tuples, the system will only see their first elements and sort accordingly. If you want your tupples sorted in a different manner, use the
key
argument to specify one.