TL;DR – Python print() can be used to show a message in the Python terminal or to write a series of characters into an external file.
Contents
What the Python print() function does
Printing in Python normally involves displaying a message or outputting a result in the Python terminal. You can use print()
with one parameter:
print(object)
However, print()
operations work with up to four parameters:
print(object(s), sep = 'separator', end = '\n', file = sys.dout, flush = False)
The objects(s)
are the objects which will be printed. The Python print() function
can take more than one of these. If multiple objects are present, then the separator
tells Python what delimiter should be inserted between each.
Meanwhile, the end
parameter allows you to insert a statement to be printed at the end of the output. file
lets you write the output to a file. Finally, flush
can forcibly output the stream following the print()
operation.
separator
, end
, file
and flush
are all keywords. Therefore, if you want to include these parameters while printing in Python, you need to pay attention to their standard use.
Here's an example of using Python print() with several parameters:
How to print in Python
It’s easy to use the print()
function in Python to print a string directly:
It’s equally easy to use print()
to display the value of an object, as shown below:
Note: print() in Python 3 was updated significantly. This guide uses print() statements for Python 3.x rather than print commands of Python 2.x.
Printing to a file in Python
If you don’t specify the file
parameter when you call the print()
command, Python will display text in the terminal.
However, if you use the open
command to load a file in write mode prior to calling the Python print()
function, characters and values can be written directly into it:
fl = open('file.txt', 'w')
print('This text will be written in the file', file = fl)
fl.close()
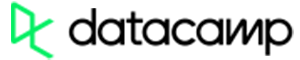
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
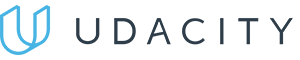
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
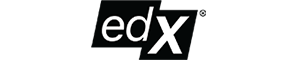
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Python 3 print() with a formatted output
A variety of characters can be used to output specially formatted text in Python. One frequently used example is %s
which will tell Python to display a numeric value as a string:
Specifying end parameters when printing in Python
You may wish to position the printed information with extra text using the end
keyword. You can also add extra strings or spaces, like in the example below where the \n
(newline) character is used:
print('This line will be three line breaks above...', end = '\n\n\n')
print('... this line of text')
The previous example code will create the output below:
This line will be three line breaks above...
... this line of text
Python print(): useful tips
- The
+
operator, which can concatenate strings in Python, also works withinprint()
operations. - The
print
statement was not a function in Python 2. In Python 3,print
became a function and requires parentheses to work.