TL;DR – The Python append method allows you to add an extra item at the end of a specified list.
Contents
The append function in Python
Lists are among the most commonly used data types in Python. They contain multiple elements: strings, numbers, dictionaries, etc. To add an extra element at the end of the list, we use the Python append
function:
You can even append Python lists with other lists:
xList = ['yellow', 'red', 'blue'];
yList = ['1', '2', '3']
xList.append(yList);
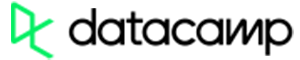
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
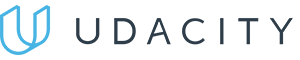
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
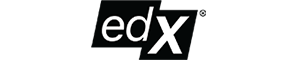
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
The syntax for append in Python
Using the Python append
method is simple – all you need to define is the list and the element to add:
list.append(element)
The append
function in Python doesn't have a return value: it doesn't create any new, original lists. Instead, it updates a list that already exists, increasing its length by one.
Python append: useful tips
- To add elements of a list to another list, use the
extend
method. This way, the length of the list will increase by the number of elements added. - To add an element to a specified location and not the end of the list, use the
insert
method. It takes two arguments: the index of the element it will be inserted after, and the new element to insert.