TL;DR – Loops are used to repeatedly execute specific instructions until a condition is satisfied.
Contents
What is a Python while loop?
A while
loop is made up of a condition or expression followed by a block of code to run. The condition or expression will be evaluated in a Boolean context. If it turns out to be true, the code within the block will be run.
This repeats until the condition evaluates as false. At this point, program execution will proceed to the first statement after the body of the loop.
As with if
statements, a while
loop can be specified on one line. If there are multiple statements in the loop body block, they can be separated by semicolons.
Python loops can have an else
clause that can be included at the end of the loop. The else
block of code runs only if the loop completes without encountering a break
statement.
Basic syntax for the while loop in Python
A while
loop in Python can be created as follows:
while <expression>:
<statement(s)>
<statement(s)>
indicates the section of code to be run with each iteration of the loop. It can also be known as the body of the loop.
Note: Python uses indentation for grouping statements: all statements indented by the same number of spaces after a construct are considered to be part of a single code block.
The controlling <expression>
will be one or more variables that are set above the loop and then updated in the body of the loop:
The output of the above code would look like this:
0
1
2
3
4
5
6
7
8
9
Before the loop begins, i
equals 0
. The expression on line 2 is i< 10
, which is true, so the body of the loop will run.
Moving into the loop body, on line 3, i
is printed and then incremented by 1
, on line 4, going from 0
to 1
.
When the body of the loop finishes processing, the script returns to the top to re-evaluate the expression. As it is still True
, the body runs again, and 2
is the output.
This process continues until i
becomes 10
. Then the expression will test as False
and the loop will end.
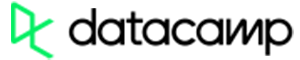
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
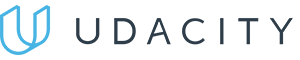
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
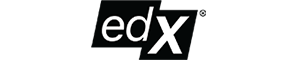
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Python break and continue statements
So far everything in the body of the loop has been run on each pass. To end the running of a while
loop early, Python provides two keywords: break
and continue
.
A break
statement will terminate the entire loop process immediately with the program moving to the first statement after the loop.
continue
statements will immediately terminate the current iteration but return back to the top. There, the control expression will be re-evaluated again to determine whether to run or end the loop.
A couple of examples will help illustrate the difference between the two:
i = 0
while i < 10:
if i == 4:
break
print(i)
i += 1
print('The end')
The above code will output:
0
1
2
3
The end
When i = 4
the break
statement runs and there is no more output.
We could also use the continue
keyword with this script:
i = 0
while i < 10:
if i == 5:
i += 1
continue
print(i)
i += 1
print('The end')
This implementation results in the output below:
0
1
2
3
4
6
7
8
9
The end
When i = 5
, the continue
statement is run and the program begins at the top again, skipping out the printing of 5
but printing the rest of the numbers in the loop.
While loop in Python: useful tips
- In Python, you can have infinite loops that are characterized by not having an explicit end.
while
loops can also be nested inside other loops.