TL;DR – In Python, not equal is a comparison operator used to determine if two variables are equal in value.
Contents
Using Python not equal
Not equal in Python is one of the comparison operators. It can have one of two return values:
True
means one variable in Python does not equal the otherFalse
means both variables are the same in value
When comparing variables, you need to take both their values and their datatypes into consideration. In the example below, you can see two integers, both with the value of 5
:
Now in this next example, you can see two variables with a value of 5
again. However, this time, A is an integer, and B is a string. As a rule, one datatype in Python does not equal a different one:
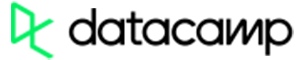
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
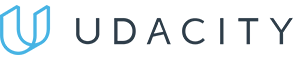
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
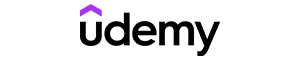
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
The syntax for not equal in Python
There are two ways to write the Python not equal comparison operator:
- !=
- <>
Most developers recommend sticking with != in Python, because both Python 2 and Python 3 support this syntax. <>, however, is deprecated in Python 3, and only works in older versions:
A != B #working
A <> B #deprecated
Python not equal: useful tips
- You can use the not equal Python operator for formatted strings (f-strings), introduced in Python 3.6.
- To return an opposite boolean value, use the equal operator ==.
- Keep in mind that some fonts change != to look like ≠!