TL;DR – The Python random module contains a set of functions for generating random numbers. The module allows you to control the types of random numbers that you create.
Contents
Why use the Python random module?
The random module can perform a few different tasks: you can use it to generate random numbers, randomize lists, or choose elements from a list at random.
This module is perfect for generating passwords or producing test datasets. It can also be integrated into Python for
or if
loops to change the outcome of a function at random.
Generating Python random integers
The most basic and common use of the random module is to generate Python random integers. To accomplish this, you will need to import the random module and then use the randint()
Python function:
This will output a random number between 0
and 10
, including end-points.
Alternatively, if you want a step size other than 1
, you can use the randrange()
function. In this case, the syntax is:
random.randrange(start, stop[, step])
The random.randrange()
function uses a step
value of 1
by default. If you specify a step
, the range of potential outputs is determined using the Python range()
function.
Generating random floating values
If you want to generate a random floating value rather than an integer, use the Python random.random()
function:
This will tell Python to generate a random number between 0
and 1
, excluding 1
.
If you want a random float number between specific start and end values, use the random.uniform()
function:
import numpy
uniform = numpy.random.uniform(0, 100, size = (3, 5))
print(uniform)
This will tell Python to generate a random float number between 0
and 100
, excluding 100
.
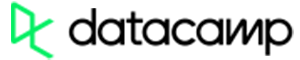
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
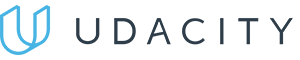
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
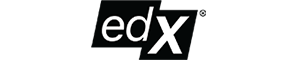
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Random functions for lists and sequences
If you have a list of numbers, values, or other elements, you can use the Python random module to randomly select one or more elements. To choose a single element at random, use the random.choice()
function:
import random
myList = ["bmw", "volvo", "toyota", "chrysler"]
print(random.choice(myList))
If you want to pick more than one element from a list or sequence, use the random.sample()
function:
import random
myList = ["bmw", "volvo", "toyota", "chrysler"]
print(random.sample(myList, 3))
In the case that you have a list or sequence and you want Python to randomize the order of elements in the list for you, use the random.shuffle()
function:
import random
myList = ["bmw", "toyota", "volvo", "chrysler"]
random.shuffle(myList)
print(myList)
Creating arrays of random numbers
If you need to create a test dataset, you can accomplish this using the randn()
Python function from the Numpy library. randn()
creates arrays filled with random numbers sampled from a normal (Gaussian) distribution between 0
and 1
.
The dimensions of the array created by the randn()
Python function depend on the number of inputs given. To create a 1-D array (that is, a list), enter only the length of the array desired. For example:
import numpy
array = numpy.random.randn(3)
print(array)
Similarly, for 2-D and 3-D arrays, enter the length of each dimension of the desired array:
import numpy
array = numpy.random.randn(3, 5, 2)
print(array)
It is possible to multiply the array generated by randn()
to get values outside of the default 0 to 1 range. Alternatively, you can use the uniform()
function to set upper and lower bounds on the random numbers generated:
import numpy
array = numpy.random.uniform(0, 100, size = (3, 5))
print(array)
Python random: useful tips
- When using
random.random()
to generate a random float number, you can multiply the result to generate a number outside the 0 to 1 range. - If you want to be able to generate the same random number in the future, check the internal state of the random number generator using the
random.getstate()
. You can reset the generator to the same state using therandom.setstate()
.