TL;DR – Python array is a one-dimensional object that allows you to store multiple elements of the same type in a single variable.
Contents
What is a Python array and why use it?
A Python array is a container that holds multiple elements in a one-dimensional catalog. Each element in an array can be identified by its respective position.
Arrays in Python can be extremely useful for organizing information when you have a large number of variables of the same type. If you had a dataset containing the names of different cities around the world, you could store them as:
car1 = "BMW"
car2 = "Audi"
car3 = "TOYOTA"
car4 = "NISSAN"
However, this would require you to keep track of which city was assigned to each variable name. You'd also have to manually enter a variable name whenever you wanted to use the name of a city within a function.
Alternatively, you could keep the city names in an array:
carsList = ["BMW", "AUDI", "TOYOTA", "NISSAN"]
Now, you can easily iterate across all of the cities in your array simply by calling the cities
variable. This becomes extremely useful when you have not three elements, but hundreds.
Python arrays vs. lists
Arrays in Python are very similar to Python lists. The main difference is that lists can contain elements of different classes, such as a mix of numerical values and text, while the elements in an array must be of the same class. A list can be considered an array if all of its elements are of the same type.
Accessing elements in a Python array
Another useful aspect of Python arrays is that you can easily find any element according to its position in the array container. For example:
carsList = ["BMW", "AUDI", "TOYOTA", "NISSAN"]
print(carsList[2])
Note: The first element in a Python array is always given an index value of 0.
You can also modify the value of any element in an array by accessing it directly:
carsList = ["BMW", "AUDI", "TOYOTA", "NISSAN"]
carsList[0] = "KIA"
print(carsList)
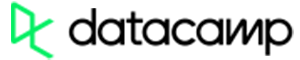
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
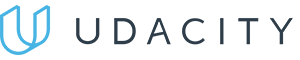
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
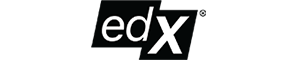
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Adding and removing array elements
You can add or remove elements from an existing Python array in several different ways.
To simply add elements onto the end of an array, use the append()
or extend()
functions. The append()
function is used when you only want to add a single element, while extend()
allows you to add multiple elements.
carsList = ["BMW", "AUDI", "TOYOTA", "NISSAN"]
carsList.append("KIA")
print(carsList)
carsList.extend(["GMC", "CADILLAC"])
print(carsList)
To remove elements from an array, use the remove()
or pop()
functions. The remove()
function takes the name of the element you want to delete and removes its first occurrence in the array. The pop()
function removes elements according to their position in the array.
carsList = ["BMW", "AUDI", "TOYOTA", "NISSAN"]
carsList.remove("BMW")
print(carsList)
carsList.pop(1)
print(carsList)
Finding the length of an array
If you need to know how big a Python array is, you can use Python’s built-in len()
function.
carsList = ["BMW", "AUDI", "TOYOTA", "NISSAN"]
print(len(carsList))
Note: the index position of the last element is equal to the length minus 1 since the first position starts at 0.
Iterating over an array
One of the most important uses of Python arrays is holding variables that you can iterate over with another function. In order to access the elements of a Python array one at a time, use a for-in
loop:
carsList = ["BMW", "AUDI", "TOYOTA", "NISSAN"]
for x in carsList:
print(x)
Python array: useful tips
- Lists are often used in place of arrays in Python because they are more flexible. However, arrays may be needed for interfacing with C code or another programming language.
- If you have multiple arrays that contain the same class of elements, you can concatenate them using the
+
operator.