TL;DR – Python global variables are declared outside a function and can be accessed anywhere in the code.
Contents
The difference between local and global variables in Python
In the example below, you can see two variables declared – bit
and bdg
:
Example
def f():
bit = "BitDegree"
print (bit)
f()
bdg = "BitDegree"
print (bdg)
While both have the same value assigned, they are declared and used differently:
bit
is declared within the function. This makes it a local variable. Its scope is the body of the function – it cannot be used anywhere else.bdg
is declared outside of the function – this makes it global. You can use Python global variables anywhere in the code.
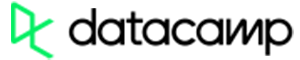
Pros Main Features
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
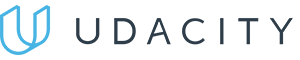
Pros Main Features
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
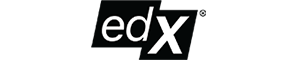
Pros Main Features
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
How to use global variables in Python
When declaring global variables in Python, you can use the same names you have already used for local variables in the same code – this will not cause any issues because of the scope difference:
- If you use the variable within the function, it will prefer the local variable by default:
- If you use the variable anywhere else in the code, you will get the value of the global variable:
Example
pizza = "Pepperoni"
def f():
pizza = "Hawaii"
print (pizza)
f()
You can also create local variables by modifying previously defined Python global variables inside the function by using the keyword global
:
Python global variables: useful tips
- You can change the value of a Python global variable by simply assigning it a new value (redeclaring).
- To delete a variable, use the keyword
del
. Trying to use a deleted variable will cause an error to fire.