TL;DR – By leaving Python comments, you can make the code more readable and simplify collaboration.
Contents
The purpose of Python comments
Like most other coding languages, Python allows the developer to comment on their code. Comments are strings of text that you can read when viewing the source code. However, they are not executed when running the code. They are mainly used to explain how the code works.
There are two main reasons to use this functionality:
- Comments allow you to understand your code when you revisit it after a while. This makes debugging and updating easier.
- A clearly commented code is easier to share and collaborate on, as it eliminates the need of explaining your lines to the colleagues.
How to leave comments in Python
Leaving a comment in Python is simple – all you need to do is add a hash mark (#
) in front of your comment:
Here you see a code line.
#here you see a comment!
A Python comment doesn't have to occupy a separate line – you can simply add it at the end of your code line. No symbols after the hash mark will be executed:
a simple code line #an inline comment
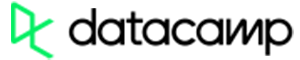
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
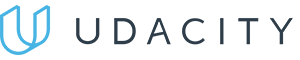
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
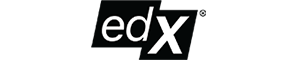
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Python comment blocks
A Python multiline comment is also known as a comment block. Unlike some other programming languages (e.g., JavaScript), Python does not have special syntax for multiline comments. Developers simply leave a few single-line comments in a row:
a code line
# a gorgeous multiline comment
# that spans over three lines
# because it needs its personal space
another code line
Another way to leave a Python comment block is by using a delimiter, which is three double quotemarks ("""
):
these are code lines
"""
this is a multiline string that belongs to no variable
because of that, Python will read it but not execute it
so it basically acts like a comment
"""
getting back to the code
However, this does not technically create a Python comment. Instead, you get a multiline string which cannot be executed because it's not assigned to any variable. The system reads the code but ignores it, so technically, it works like a Python multiline comment. Most developers don't recommend this practice for beginners, as it can potentially cause errors.
Python comment: useful tips
- You can comment out Python code when testing or debugging. Add a hash mark in front of a line to disable it temporarily, and then run the code to see whether the bug still occurs. This way, you can easily find the exact section of the code that causes the issue.
- There are tools (e.g., pdoc and pydoc) that allow you to generate documentation from the multiline docstrings in your code.