TL;DR – Python class is a blueprint for a kind of object. In Python, you can instantiate classes to create new objects.
Contents
What is a Python class?
Unlike a procedural programming language, any coding done in Python revolves around objects. In some object-oriented languages, objects are just basic chunks of data and attributes. However, in Python, they consist of functions as well as information.
In short, a Python class is for defining a particular type of object. Because Python objects can have both function and data elements, Python classes define what methods can be used to change the state of an object. They also indicate what attributes the object can have.
Creating a Python class definition
It’s not hard to define Python class. To do so, you’ll need the class
keyword:
class Example:
"A basic example class"
variable = 123
If you run the above code in a Python environment, you’ll find you can call Example.a
to return an integer value. This is an example of a class for data-only objects, but it’s equally easy to define a class that returns a function object by adding the def
keyword to your code:
class Example:
"A basic example class that returns a function object"
def b(self):
return "this is an example class"
Instantiating a Python class object
With the above Python class examples, you can create a class object through attribute referencing using Example.a
and Example.b
respectively.
It’s also possible to instantiate a class object via function notation. Simply set a variable equal to the class in the same way you’d call a function with no parameters:
class Example:
"A basic example class that returns a function object"
def b(self):
return "this is an example class"
c = Example()
With this, a new class object will be instantiated and attributed to local variable c
.
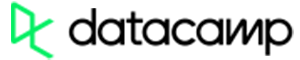
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
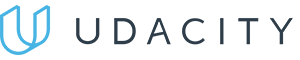
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
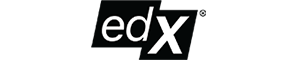
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
The constructor method for class object instantiation
It’s possible to simulate the constructor of a class in Python using the __init__()
method. This is a helpful way to initialize attributes for all objects in a given class:
class Square:
def __init__(self, length, width):
self.length = length
self.width = width
def area(self):
return self.width * self.length
r = Square(20, 2000)
print("Rectangle Area: %d" % (r.area()))
This Python class example results in the following output:
Rectangle Area: 40000
Note: Python uses a default constructor for any class where you don’t add your own.
Working with complex class objects
Of course, a key reason to use classes in Python is that doing so allows you to create custom object types. With these, you can instantiate variables that represent things like co-ordinates or other complex data points:
class PartsColor:
"Creates a class"
def __init__(self):
"Create a new color for each part"
self.hood = "Blue"
self.wheels = "Red"
self.doors = "Green"
e = PartsColor() # Instantiate two objects to represent points
f = PartsColor()
print(e.hood, f.wheels, e.hood, f.wheels, e.doors, f.doors) # Assign each point a unique location
This will output:
Blue Red Blue Red Green Green
Python class: useful tips
- You can use Python functions like
getattr(obj,name,default)
to check and modify the attributes of an object even after they have been initialized through a Python class. - In Python classes, there’s a big difference between inheritance and composition. Inheritance transfers attributes and methods used in one class to another. Composition means that a base class isn’t inherited from.