TL;DR – Functions are reusable blocks of code that allow you to pass specific input parameters to them. With Python functions, you can execute a set of code without having to re-enter it.
Contents
Why use functions in Python?
The main reason to use Python functions is to save time. You might need tens of lines of code to perform one or more tasks on a set of inputs. However, you can simplify the task by defining a function in Python that includes all of them.
Note: next time you need to execute that task, you can use the function and simply define your inputs rather than re-enter long code.
On top of that, functions are easily reusable. Code contained in functions can be transferred from one programmer to another. This eliminates the chance of losing lines of code or incorrectly entering the code underlying the function.
Tip: Python has thousands of built-in functions such as print(), abs(), and range(). Plus, modules and libraries act like packages of additional functions for you to use.
Defining functions in Python
To define a function in Python, use the def
command followed by the name of your new function:
def myFunction():
print("This is my new function")
To call or execute your function, simply enter its name followed by parentheses:
def myFunction():
print("This is my new function")
myFunction()
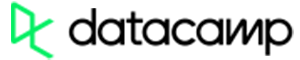
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
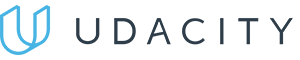
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
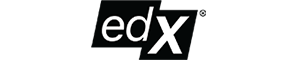
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Adding input parameters to functions
If you want to add parameters that can be passed to the function as inputs, you will add them when defining the function.
Name your input variables inside the parentheses, separated by commas, and reference them in the code underlying the function. For example:
def myInfo(name, age):
print("My name is %s and age %d" % (name, age))
myInfo("Paul", 20)
Note: Python returns an error message if you pass inputs that are not supported by your function.
This Python function example requires you to specify inputs for both the month and day parameters. You can set default values for both parameters when defining the function:
def myInfo(name="Paul", age=20):
print("My name is %s and age %d" % (name, age))
myInfo()
Now, if you call the myBirthday()
function without specifying any inputs, Python will use the default values of February and 10 for the month
and day
arguments.
You can specify default values for any number of parameters in a Python function. However, once one parameter has a default value defined, the Python function syntax requires that every next parameter in the function definition would also have a default value.
You can change the order in which parameters are entered when executing a function if you call them by their parameter names. As an example:
def myInfo(name="Paul", age=20):
print("My name is %s and age %d" % (name, age))
myInfo(age=23, name="Joe")
Python functions: useful tips
- It is possible to define variable length functions in which the number of input parameters is undefined. To do so, define your function with an asterisk (
*
) before the variable name and pass a list of values as inputs to your function. - When defining function names and input parameter names, be careful that you do not overwrite built-in Python functions or functions imported from a module.