TL;DR – Python enumerate() is a built-in function that allows you to loop through iterable type objects.
Contents
How enumerate() works
The enumerate()
function assigns an index to each item in an iterable object that can be used to reference the item later. What does enumerate
do in Python? It makes it easier to keep track of the content of an iterable object.
If you are using enumerate
in Python 3 and call print(enumerate())
, you will get an alphanumeric memory reference address instead of a readable list. enumerate()
in Python 3 works this way to save memory by not generating unnecessary full-fledged lists.
Basic syntax of Python enumerate()
To call enumerate()
in Python, you will need to provide the function with two arguments.
enumerate(iterable, start=0)
In the example above, iterable
is an iterable type object like a list, tuple, or string.
start
is an optional parameter that tells the function which index to use for the first item of the iterable
.
Examples how enumerate() can be used
If you don’t provide a start
parameter, enumerate()
will use a start
value of 0
by default.
cars = ['kia', 'audi', 'bmw']
for car in enumerate(cars):
print(car)
However, if you provide a non-zero start
value, Python will begin enumerating from it, as demonstrated below:
cars = ['kia', 'audi', 'bmw']
print(list(enumerate(cars, start = 1)))
Working with enumerate() in Python
The enumerate()
function in Python is commonly used instead of the for
loop. That’s because enumerate()
can iterate over the index of an item, as well as the item itself.
Using enumerate()
also makes the code cleaner, since you have to write fewer lines. For example, recall the code snippet we previously used:
cars = ['kia', 'audi', 'bmw']
print(list(enumerate(cars, start = 1)))
Using a for
loop to do the same thing as the code above would look something like this:
cars = ['kia', 'audi', 'bmw']
listOfCars = []
n = 0
for car in cars:
listOfCars.append((n, car))
n += 1
print(listOfCars)
This code isn’t just longer. You can also see that a complex for
loop implementation would get clunky and error-prone easily.
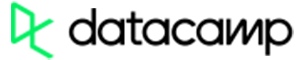
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
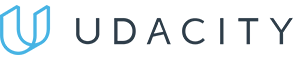
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
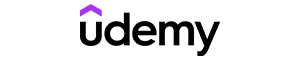
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Working with tuples
As mentioned above, you can enumerate over a tuple as well since tuples are iterable objects. Doing so is very similar to enumerating over a list:
Working with strings
In Python, strings are iterable. That means you can use enumerate()
with them the way you would with any other iterable object:
Looping through an object with enumerate()
Let's say that you have an object that you want to enumerate in Python, and get each component individually. You can loop through the object to do so.
cars = ('kia', 'audi', 'bmw')
for car in enumerate(cars):
print(car)
Python enumerate(): useful tips
- By default, the Python
enumerate()
function returns an enumerated object. You can convert this object to a tuple or a list by usingtuple(<enumerate>)
andlist(<enumerate>)
, respectively. - Using
enumerate()
instead of more verbose loops not only makes the code cleaner but is a more Pythonic way of programming. Such loops can be messy and can have more errors.