TL;DR â A Python set is a built-in data type that stores a group of elements without ordering them. No duplicate elements are allowed in a given set.
Contents
Defining a set
You can define a set by using the Python set()
function. To use it, youâll need to provide the elements you want to include in the set in the form of an iterable object such as a string.
There are two types of sets in Python: set
and frozenset
. The difference between the two is that a set
is mutable, which means that you can change its contents, while a frozenset
is not. Because a frozenset
canât be changed once created, you can only use operations that donât modify set contents on a frozenset
.
Note: in Python, strings, lists and tuples are all iterable.
Alternatively, you can define a set explicitly using curly braces {}
. When you define a set in this manner, each element you add is treated as a distinct element of the set:
yourSet = {'bmw', 'toyota', 'volvo', 'chrysler'}
Note: Python sets can be modified at any time after creation.
Creating an empty set
You can define an empty set using the set
function:
yourSet = set()
print(yourSet)
You cannot, however, define an empty set using curly brackets ({}
) since Python interprets a set of empty curly brackets as a dictionary:
Set elements
Python sets can include objects of different types, as long as they cannot be modified. That means you canât include data types such as dictionaries:
yourSet = {122, 'toyota', 2.66, (3, 2, 1), True}
print(yourSet)
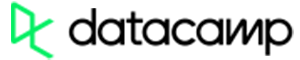
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
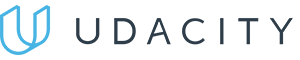
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
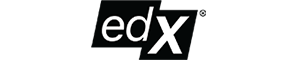
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Getting information about the set
In Python, you can retrieve the length of a set as follows:
Itâs also easy to determine if an element is a member of a set or not:
yourSet = {'bmw', 'volvo', 'toyota'}
print('bmw' in yourSet)
print('chrysler' in yourSet)
Methods and operators for sets
Below is a list of available Python set operations. If you have two sets (one called set1
and another called set2
), you can perform joint operations on both sets by either using an operator or calling a method function. Any exceptions are noted in the following table.
Set Operation | Returns... | Operator | Method |
---|---|---|---|
Union | All unique elements in set1 and set2 |
| | union() |
Intersection | Elements present in set1 and set2 |
& | intersection() |
Difference | Elements that are present in one set, but not the other | - | difference() |
Symmetric Difference | Elements present in one set or the other, but not both | ^ | symmetric_difference() |
Disjoint | True if the two sets have no elements in common |
None | isdisjoint() |
Subset | True if one set is a subset of the other (that is, all elements of set2 are also in set1 ) |
<= | issubset() |
Proper Subset | True if one set is a subset of the other, but set2 and set1 cannot be identical |
< | None |
Superset | True if one set is a superset of the other (that is, set1 contains all elements of set2 ) |
>= | issuperset() |
Proper Superset | True if one set is a superset of the other, but set1 and set2 cannot be identical |
> | None |
Modifying sets
If you need to modify individual sets, you can also do so by performing set operations. Python allows you to:
- Add an element to a set:
yourSet.add(<element>)
- Remove an element from a set:
yourSet.remove(<element>)
- Remove an element from a set if present; otherwise, nothing occurs:
yourSet.discard(<element>)
- Remove a random element from a set:
yourSet.pop(<element>)
- Clear a set by removing all elements:
yourSet.clear()