TL;DR β CSS alignment is an important part of creating layouts for web design. There are a number of ways to align elements horizontally or vertically.
Contents
- 1. CSS horizontal align
- 1.1. Aligning inline elements
- 1.2. Using margins for block elements
- 1.3. Modifying position
- 1.4. Making elements float
- 2. CSS vertical align
- 2.1. Inline elements and vertical-align
- 2.2. Centering with padding
- 2.3. Aligning with line-height
- 2.4. Combining position and transform
- 3. CSS align: useful tips
CSS horizontal align
Aligning inline elements
Inline elements and text are the easiest to align. You can do that by using the text-align property:
This property can take one of six possible values:
Value | Syntax | Description |
---|---|---|
center |
text-align: center; |
The default value. Makes CSS center the element in the container |
left |
text-align: left; |
Makes CSS align the element at the left side of the container |
right |
text-align: right; |
Makes CSS align the element at the right side of the container |
justify |
text‑align: justify; |
Makes CSS space the content to fill the container from edge to edge |
start |
text-align: start; |
Works like left when the text direction is left-to-right and like right when it's right-to-left |
end |
text-align: end; |
Works like right when the text direction is left-to-right and like left when it's right-to-left |
Using margins for block elements
To make CSS center or otherwise align a block element, set both its left and right margins to auto
. See how to center div elements in the example below:
.center{
margin: auto;
padding: 15px;
border: 4px solid black;
width: 88%;
}
Note: for this to work, an element has to have a set width.
To be able to do the same to any element, you can apply a display property with its value set to block
to it. For example, to center an image horizontally, you would follow the example below:
img {
display: block;
margin-right: auto;
margin-left: auto;
width: 60%;
}
Tip: to place multiple block elements in one row, apply the
display
property with its value set toinline-block
to them.
Modifying position
If you only have one element in the container, you can also align it horizontally by using the position property. If you define the absolute
value, the position will be fixed, which means that the element may overlap other elements to keep its particular place when a user resizes the window or scrolls:
.left{
left: 15px;
position: absolute;
border: 4px solid black;
padding: 15px;
width: 550px;
}
Note: if you have multiple elements in one container, the absolute position will be applied to all of them.
Making elements float
You can also align elements using the float
property:
.left {
float: left;
border: 4px solid black;
padding: 15px;
width: 550px;
}
The float
property is different from absolute position in two aspects. First, it places an element on the specified side of the container so that text and other inline elements can wrap around it.
Secondly, the floated element is removed from the normal flow of the page. However, it does remain a part of the flow in a sense that other elements donβt ignore it completely (which they do for absolutely positioned elements).
When you float an image or another element that has a bigger height than its container, such element overflows the boundaries of the container. You can prevent this by applying the overflow property with its value set to auto
:
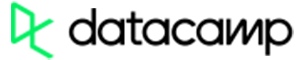
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
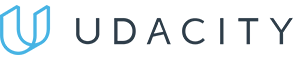
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
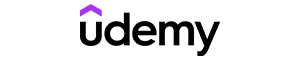
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
CSS vertical align
Inline elements and vertical-align
The vertical align CSS method can be used for inline elements:
img.first {
vertical-align: baseline;
}
img.second {
vertical-align: text-top;
}
img.third {
vertical-align: text-bottom;
}
img.fourth {
vertical-align: sub;
}
img.fifth {
vertical-align: super;
}
Note:
vertical-align
will also work on table cells and elements that have theirdisplay
property set totable-cell
.
Centering with padding
Just like margins can be used for horizontal alignment, padding can help you center elements vertically. All you need to do is apply equal-sized padding on the top and the bottom of the element:
This option is great when you aren't sure about the exact dimensions of the element and therefore cannot count where exactly is the middle.
Note: make sure to define the right and left padding as
0px
: if you only specify one value forpadding
, CSS will use it for all four sides of the element.
To center text both vertically and horizontally, you can combine padding
with text-align: center
:
.center {
padding: 30px 0;
border: 4px solid black;
text-align: center;
}
Aligning with line-height
You can also center an element vertically by setting the line-height value to be identical as the height
of the element. This gives a similar effect as setting equal padding:
.center {
border: 4px solid black;
text-align: center;
line-height: 100px;
height: 100px;
}
/* Add the following in case the text has more than one line: */
.center p {
line-height: 1.5;
display: inline-block;
vertical-align: middle;
}
Have in mind though that this method is only suitable when you have a single line of text to center. However, this method may work better for specific fonts, as it sets the baseline correctly. That ensures the text appears to be precisely in the middle. Still, this argument is only valid when you set it using pixel values.
Combining position and transform
One more way to vertically center an element is by using position
and transform together. There may be situations where neither padding
nor line-height
are suitable options β e.g., if your container has a fixed width and multiple lines of text:
.center {
border: 4px solid black;
height: 100px;
position: relative;
}
.center p {
margin: 0;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
CSS align: useful tips
- You'll find it easier to align elements if you learn to work with Flexbox.
- If you want a group of elements to go together and be aligned as a unit, group them by wrapping them in the same container. E.g., you can center div containers as your usual blocks.