In our introductory tutorial, we mentioned that basic knowledge of coding languages might help learn jQuery, too. This is exactly the case with jQuery selectors: if you know at least a little bit of CSS, you are also familiar with the CSS selectors.
The jQuery library uses both CSS and its unique selectors. As the name itself suggests, the jQuery selector is used to select elements. By choosing particular relevant criteria, you may choose certain HTML elements that you will then apply a function to.
In this tutorial, we will introduce you to the jQuery selectors you might use and explain how to combine them to get more specific results. Examples will follow the theory so you can grasp the concept in no time.
Contents
jQuery Selector: Main Tips
- jQuery borrows from CSS, utilizing the selectors, as well as adding its own, which are used for matching a set of elements from the HTML DOM.
- Selectors in jQuery are meant to specify a set of elements based on certain attributes, such as ID, class, or the type of tag itself.
- These elements can then be selected for applying the jQuery method or a function you define.
How it Works
To begin with, all selectors in jQuery start with a $ symbol - a lot like this example, which will select every element with the <button> tag in the document:
$("button")
Once we have selected the set of elements, we can apply the method or function of your choice. For example, if we would like buttons to become blue on click, this is the code we would use to do that:
This particular selector would be referred to as an element selector. In addition to this one, there are many others that select elements based on different conditions.
Although many of the meta characters are used as selectors, you can include them in the values of class and ID attributes when selecting as well. However, they must be escaped using to backslashes before the character. For example, if you wanted to select an element with the id attribute with the value nav.bar, the selector would be $("nav//.bar")
and not "$("nav.bar")
".
Combining Selectors
It is also possible to combine multiple selectors in jQuery. To include more than one selector in a jQuery statement and get the elements of all of them selected, you simply need to separate them by commas like this:
$("selector1, selector2, selectors3, ..., selectorn")
When selecting multiple attributes, however, you do not need to use the commas to separate them. You may simply place the jQuery attribute selectors one after another:
$("[attribute1='value'][attribute2='value'][attribute3='value']")
Basic Types
We'll start by introducing the most basic selectors you have available. Let's get familiar with their purpose and standard syntax.
this
is used to select the current element - the one that the jQuery code is inside directly:
$("this")
*
(the asterisk symbol) when used as a selector, simply selects all of the elements inside a document:
$("*")
$(document).ready(() => {
$("*").css("background-color", "green");
});
Now, there are two selectors that are mainly used to select very specific items based on their id and class attribute values. The main difference between them is the amount of elements they select. Keep in mind that id is exclusive to a single element:
$("#id")
Now, class
is more commonly used on a specific group of elements. Therefore, jQuery class selector is useful when, for example, you want to apply a certain style on those elements:
$(.class")
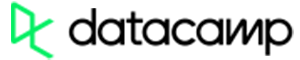
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
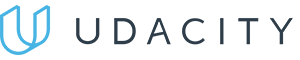
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
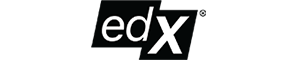
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Selecting by Attribute Value
jQuery has a plenty of selectors that select HTML elements with attributes that meet certain conditions. They are commonly referred to as jQuery attribute selectors.
See the comprehensive list below, and read the descriptions. Dedicate some time to them, and you get familiar with the jQuery attribute selectors in detail:
$("[attribute]")
- selects a set of elements that have the specified attribute.("[attribute='value']")
- selects a set of elements that have the specified attribute with the specified value.$("[attribute!='value']")
- selects a set of elements that do not have the specified attribute with the specified value.$("[attribute|='prefix']")
- selects a set of elements that have the specified attribute with the value that has a specified prefix (separated from the rest of the value name by a hyphen).$("[attribute*='value']")
- selects a set of elements that have the specified attribute with the value that contains the specified substring. A substring can be a part of another string anywhere inside it, so the phrase you specify as the value does not have match the whole.$("[attribute$='value']")
- selects a set of elements that have the specified attribute with the specified value at the end.$("[attribute^='value']")
- selects a set of elements that have the specified attribute with the specified value at the start.
$(document).ready(() => {
$("[id]").css("background-color", "green");
});
Parents vs. Children
Another type of selectors that are important to note is those that select elements based on their hierarchical relationship. They are commonly referred to as jQuery child selectors or parent selectors. The list below describes them and what they do:
$("parent>child")
- combines two selectors: a jQuery child selector and a parent selector. They select the elements specified as child - ones that are children of elements specified as parent.$("ancestor descendant")
- combines two selectors to select all elements specified as descendant - ones that are below the elements specified as ancestor in the node relationships.$(":root")
- selects the document's root element.$(":parent")
- selects elements that have at least a single child node.$(":empty")
- selects all elements that have no children (this would include text nodes as well).
$(document).ready(() => {
$("td:parent").css("background-color", "red");
});
The Keyword-Based Types
Some jQuery selectors are keyword-based. You may recognize them easily, as they are always preceded by a colon (:).
In this section, we will cover a few different types of such selectors. Review the lists carefully and get to know them. Understanding these selectors will help you use jQuery much more effectively.
Element Type Selectors
These selectors are meant to select elements that have a certain type or certain tags:
$(":button")
- selects button elements and elements that have the type attribute with the value button.$(":radio")
- selects radio elements.$(":checkbox")
- selects all checkbox elements.$(":checked")
- selects all selected or checked elements.$(":disabled")
- selects all disabled elements.$(":file")
- selects all element of the file type.$(":submit")
- selects elements of the submit type.$(":header")
- selects header elements (h1, h2, h3, h4, h5, h6).(":image")
- selects all image elements.$(":input")
- selects all input, select, textarea and button elements.$(":text")
- selects all elements of text type.$(":reset")
- selects all elements of reset type.
$(document).ready(() => {
$(":radio").wrap("<span style="background-color: green;"></span>");
$(":checkbox").wrap("<span style="background-color: green;"></span>");
});
Nth-Of-*, Only, First and Last Selectors
These selectors are meant to select elements based on their type, node relationships, and the document layout:
$(":nth-child(n)")
- selects the nth children of the specified parent elements.$(":nth-last-child(n)")
- selects the nth children of the specified parent elements, counting from last child to the first.$(":nth-last-of-type")
- selects the nth children of the specified parent elements, in relation to siblings of the same element name, counting from last child to the first.$(":nth-of-type")
- selects the nth children of the specified parent elements, in relation to siblings of the same element name.$(":first-child")
- selects all elements that are the first child of their parent element.$(":first-of-type")
- selects all elements that are the first among other elements of the same type.$(":first")
- selects the first element of the matched type.$(":last-child")
- selects the element that is the last child of the specified parent.$(":last-of-type")
- selects all elements that are the last of their type.$(":last")
- selects the last element matched.$(":only-child")
- selects elements that are the only child of their parents.$(":only-of-type")
- selects elements that are the only ones of their type.$(":eq()")
- selects the nth element in the matched set.
$(document).ready(() => {
$("p:nth-child(3)").css("background-color", "green");
});
Other Selectors
As always, everything cannot be neatly organized. Some jQuery selectors, too, have purposes that don't allow them to be grouped with others.
The selectors in the list below have various uses, which do not fall under any of the categories listed above. They aren't any less useful, so make sure to review them carefully:
$("prev + next")
- selects the next element adjacent to the element specified as prev, which matches the type specified by selector next.$("prev ~ siblings")
- selects the siblings following the element specified as prev, which match the type specified by selector sibling.$(":animated")
- selects all elements that are being animated at the moment.$(":enabled")
- selects all elements that are enabled.$(":contains('text')")
- selects all elements containing the text specified.$(":even")
- selects even, zero index elements.$(":odd")
- selects odd, zero index elements.$(":focus")
- selects the element that is currently focused.$(":gt()")
- selects the elements whose index is greater than that of the currently matched set.$(":has(selector)")
- selects the elements that have the specified element among any of its descendants.$(":hiden")
- selects elements that are hidden.$(":lang()")
- selects all elements of the specified language attribute value.$(":lt()")
- selects the elements whose index is less than that of the currently matched set.$(":not")
- selects the elements that do not match the specified selector.$(":selected")
- selects the selected elements.$(":target")
- selects the target element specified by the document's URI.$(":visible")
- selects all elements that are visible.
$(document).ready(() => {
$("tr:gt(3)").css("background-color", "red");
});
jQuery Selector: Summary
- The selectors jQuery uses are both borrowed from CSS and unique.
- These selectors are used to look for matches of a certain criteria and select a set of HTML DOM elements.
- ID, class, or the type of tag itself can be chosen as such criteria.
- Selecting a certain set of elements makes it easier to apply selected functions to them.