Contents
jQuery mouseover: Main Tips
- The
.mouseover()
method in jQuery can either trigger amouseover
event, or attach an event handler to it. - The
mouseover
event occurs when the cursor enters an element. - While this method works very similarly to .mouseenter(), it reacts to event bubbling (
.mouseenter()
does not).
.mouseover() Method Explained
The jQuery .mouseover()
method attaches an event handler, running a function when the mouseover
event occurs. The method can also trigger the jQuery mouse over event.
In the example, the background color of the paragraph changes to red as you move the cursor over it:
$("p").mouseover(() => {
$("p").css("background-color", "red");
});
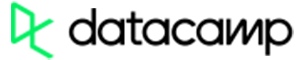
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
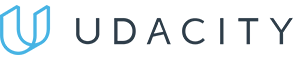
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
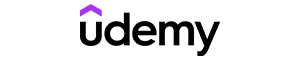
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Syntax for .mouseover() in jQuery
To trigger a mouseover
event manually, use the jQuery .mouseover()
method without any arguments:
$("selector").mouseover();
To attach an event handler, you will have to include at least one parameter:
$("selector").mouseover(data, callback);
The callback parameter defines the callback function that you wish to attach as the event handler. If the function you chose needs some data
to execute properly, you can add it in the brackets before specifying the function.
Note: when adding an event handler with jQuery .mouseover(), remember that it is a shortcut for .on( "mouseover", callback). Thus, you can remove the handler simply by using .off().
jQuery .mouseover() or .mouseenter()?
A common issue beginners face is understanding the difference between jQuery .mouseenter()
and .mouseenter(). You won't see any difference using them on elements with no descendants. However, when elements do have children, these methods behave differently:
var i = 0;
$( "div.overout" )
.mouseover(function() {
$( "p:first", this ).text( "mouse over" );
$( "p:last", this ).text( ++i );
})
.mouseout(function() {
$( "p:first", this ).text( "mouse out" );
});
var n = 0;
$( "div.enterleave" )
.mouseenter(function() {
$( "p:first", this ).text( "mouse enter" );
$( "p:last", this ).text( ++n );
})
.mouseleave(function() {
$( "p:first", this ).text( "mouse leave" );
});
The difference is caused by event bubbling, which jQuery .mouseover()
supports and .mouseenter()
does not. Event bubbling means that when an event occurs, its handlers are executed on the specified element first and then run on its parent (and other ancestors, if found).
Note: while the CSS :hover selector might seem like a similar option, it does the job of both .mouseover() and .mouseout() at once: the applied effect disappears after you move the cursor out.