You can create custom animations by using jQuery animate method. In this jQuery animate tutorial, we will explain the standard syntax and the options provided by the different parameters it takes. You will also learn to create animation sequences.
Contents
jQuery Animate: Main Tips
- The jQuery animate method is used to animate the CSS values of an object.
- Before you use animate in jQuery, you need to make sure particular values are animatable.
The Method
Animate in jQuery is the most versatile method for applying effects to object. It is not difficult to use as long as you are familiar enough with CSS. This method can be used in various ways, accepting unlimited parameters which specify what CSS properties should be affected, as well as the duration and a callback function (which are both optional).
The syntax pattern for jQuery animation effects is as follows:
$(selector).animate({parameters},duration,callback);
Let's review each of the syntax elements used for animate in jQuery:
- parameters is the most important part of the animate jQuery method. The parameters are the CSS properties to be animated. They can be absolute or relative values, and the properties should be animatable.
- duration defines a string or number value that specifies how long (in milliseconds) the animation will last.
- callback specifies the function that will be called once the method's is executed.
Of course, jQuery animation examples help understand the topic much better than theory. Here we have a basic one to demonstrate how the animation can be used:
In this example, the element #animatedItem will stretch to 200px height in 400 milliseconds, which is the default animation length.
Note: color animations are not a part of the jQuery library, but this can be fixed with the color animations plugin you can find at http://plugins.jquery.com/.
It should be noted that animation can apply to any number of properties at once. Commas separate the different properties with their values (which are surrounded with single quotes). Look at this example to see how it could look like in actual code:
$("button").click(() => {
$("div").animate({
left: '500px',
opacity: '0.25',
height: '250px',
width: '100px'
});
});
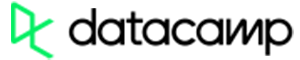
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
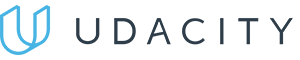
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
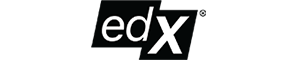
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Animation Sequences
To create more complex animations you can try to apply multiple animations in a sequence. It is not hard to do: first, you have to assign the element you would like to queue animations for to a variable like this:
var identifier = $(selector);
Next, you use this variable to refer to the element you would like to animate in jQuery, and apply the animate method as many times as you like. See the jQuery animation examples below:
$("button").click(() => {
var div = $("div");
div.animate({left: '100px'}, "slow");
div.animate({fontSize: '4em'}, "slow");
});
Relative and Predefined Values
It is possible to modify the CSS properties using relative values. To make the value you assign via the animate jQuery method relative, simply add += to add the value you assign to the pre-existing value and -= to subtract. This is how it would look like in actual code:
$("button").click(() => {
$("div").animate({
left: '225px',
height: '+=100px',
width: '+=170px'
});
});
Note: this only works on numerical values.
You can even specify a property's animation value as show, hide, or toggle:
$("button").click(() => {
$("div").animate({
width: 'toggle'
});
});
jQuery Animate: Summary
- You may use a method called animate in jQuery to animate an object's CSS values.
- The values you choose should be animatable for you to apply the jQuery animation effects.