Learning any language begins with the very basics, and jQuery is no exception. One of the first concepts you should have grasped by now is jQuery events. Simply put, any user interaction can be called an event.
In this chapter, we will be covering various jQuery event methods you can use. They will be grouped into categories according to the type of events they are used on. These methods are mostly used to add, remove, or modify jQuery event handlers.
jQuery Event Methods: Main Tips
- jQuery offers a bunch of special methods you may use when handling various user interaction events.
- Most of them make jQuery add event listeners, or handlers, to a certain event.
- jQuery event methods are usually categorized by events they are directly related to.
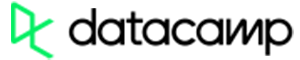
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
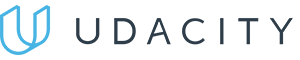
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
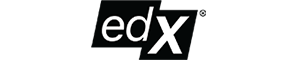
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Methods Explained
First, we will introduce you to all the jQuery event methods used to set, remove and manipulate event handlers. See the table below and memorize the functions:
Method | Description |
---|---|
.bind() | Adds a jQuery event handler to fire when an event of a defined type is sent to the element. |
.delegate() | Adds a jQuery event handler (or multiple) to fire when an event of a defined type is/are sent to a descendant element matching selector. |
jQuery.proxy() | Takes an existing function and returns a new one that will always have a particular context. |
.on() | Attaches jQuery event handlers to elements selected. |
.off() | Makes jQuery remove event handlers added using the on(). |
.one() | Attaches 1≤ jQuery event handlers to elements. Run once per element at most. |
.trigger() | Executes all handlers and behaviors attached to elements for the given event type. |
.triggerHandler() | Executes all handlers attached to elements for an event. |
.unbind() | Makes jQuery remove event handler already attached. |
.undelegate() | Removes the event handler bindings on the element made using delegate(). |
.on()
is probably the most common method - see how it works:
$("div").on("click", function() {
$(this).css("background-color", "red");
});
Most of the other functions are grouped according to the events they are related to. For example, the jQuery mouse event methods are used to work with mouse events, such as clicks or hovers:
Method | Triggers or adds a jQuery event handler to fire when |
---|---|
.click() | The element is clicked. |
.dblclick() | The element is double-clicked. |
.hover() | The mouse pointer moves in and out of the elements. Might add 1 or 2. |
.mousedown() | The mouse button is pressed inside element. |
.mouseenter() | The mouse moves into element. |
.mouseleave() | The mouse moves out of element. |
.mousemove() | The mouse pointer moves inside element. |
.mouseout() | The mouse pointer moves out of element. |
.mouseover() | The mouse pointer moves into element. |
.mouseup() | The mouse button is released inside element. |
See a simple code example of a click event below:
$(document).ready(() => {
$("p").click(() => {
alert("The paragraph was clicked!");
});
});
Note: Previously, .toggle() could have been used to add 2≤ handlers to selected elements, to be executed on alternate clicks. However, it was deprecated in v1.8.
Now, keyboard events relate to the actions users cause by using the keyboard of their device, like pushing a button. There are only three of them, so they'll be easy to remember:
.keydown()
triggers or adds an event handler to fire when a key is pressed..keypress()
triggers or adds an event handler to fire when a keystroke occurs..keyup()
triggers or adds an event handler to fire when a key is released.
See the usage of .keypress()
in a simple code example below:
var i = 0;
$("input").keypress(() => {
$("p").text(i += 1);
});
Note: For these methods to work, the defined element must have a keyboard focus.
There are seven jQuery event methods specially meant for handling the form elements’ actions. See them in the table below:
Method | Triggers or adds a jQuery event handler to fire when. |
---|---|
.blur() | The element loses keyboard focus. |
.change() | The value of the element changes. |
.focus() | The element gains keyboard focus. |
.focusin() | The element (or its descendant) gains keyboard focus. |
.focusout() | The element (or its descendant) loses keyboard focus. |
.select() | Text is selected in element. |
.submit() | The form element is submitted. |
See how a simple .focusin()
function works:
$("input").focusin(function() {
$(this).css("background-color", "red");
});
The last group of jQuery event methods is meant to be used with document and window events. Currently, only three of them are relevant, as a few have been deprecated by v1.8. Let's first review the ones we might use currently:
.ready()
adds an event handler to fire when the DOM finishes loading..resize()
triggers or adds an event handler to fire when the size of the element is changed..scroll()
triggers or adds an event handler to fire when the scroll position of the window or element is changed.
The deprecated ones include:
.error()
, which was used to add an event handler to fire when the DOM was not loaded properly..load()
, which was used to add an event handler to fire when the HTML element finished loading..unload()
, which was used to add an event handler to fire when the user navigated away from the page.
jQuery Event Methods: Summary
- Handling jQuery events can be simplified by using special methods. Using them, you can easily make jQuery add event listeners to defined events.
- jQuery event methods are easy to work with, as they are categorized by the type of events they relate to.