A URL redirect takes the user to a different URL from the one they typed in or clicked on. It is often used for website restructures or during maintenance.
Contents
- 1. Server-side vs. client-side
- 2. 301 or 302: redirect codes explained
- 3. How to create a server-side redirect
- 3.1. Apache web server: the .htaccess redirect
- 3.2. Including URL redirects in PHP header function
- 3.3. Using url.redirect in Lighttpd
- 3.4. The scheme variable in Nginx
- 3.5. The redirect function in Flask
- 3.6. For the Ruby coders: redirects in Rails
- 4. How to redirect a URL on the client side
- 4.1. Meta redirects: with or without delays?
- 4.2. Using the JavaScript window.location object
Server-side vs. client-side
All types of URL redirects can be divided into two groups:
- Server-side: the server responds to the browerâs request with a 30X status code
- Client-side: the browser itself makes the redirect (can be done with HTML or JavaScript)
Note: in a case of multiple redirects of different types, the server-side redirects will execute first. HTML redirects will also happen before those written in JavaScript.
301 or 302: redirect codes explained
Every time you try to access a certain URL, your browser sends a GET request to the server. The server replies with a certain status code which is a three-digit number. A status code can represent a successful response as well as an error.
For URL redirects, the first digit of the status code is always 3. In the table below, you can see all the choices explained with examples.
Status code | Meaning | Explanation |
---|---|---|
301 | Moved Permanently | The content has been moved for good and all future requests should be directed to the new URL |
302 | Found | The content has been moved temporarily and all future requests should be directed to the original URL |
303 | See Other | A form found on the page cannot be resubmitted by using Back. All future POST requests are changed to GET |
307 | Temporary Redirect | The HTTP 1.1 version of the 302 redirect |
308 | Permanent Redirect | The HTTP 1.1 version of the 301 redirect |
In most cases, we recommend you to use simple 301 redirects. It is the only redirect type which passes the link authority, also known as link juice. The more pages have links to your page, the more link juice it collects, and the higher it ranks on search engines. Therefore, you need it transferred to the new URL as well.
The 302 redirect passes no link juice because itâs meant for temporary redirects only. You donât want to lower the ranking of the original URL, because you will use it again in the future. The browser also doesnât cache 302 redirects by default.
Website owners usually use 302 redirects for things like special offers that repeat after some time. When the offer is no longer valid, you can redirect its visitors to the landing page of your website. However, when you want to offer the deal again, you can reclaim its URL with the same SEO value it had before.
Note: always try your best to avoid redirect chains which occur when one redirect leads to another and so on. This slows down loading, plus, you lose small amounts of link juice with every new redirect.
How to create a server-side redirect
There are multiple ways you can write a server-side redirect, and the syntax requirements differ in each web server. Weâre going to discuss the most popular ones with examples in the following sections.
We will mostly be using 301 redirects in our examples, as itâs the most common type. However, you are free to use any status code as needed.
Apache web server: the .htaccess redirect
One of the most common ways to create a URL redirect is adding a rule to .htaccess. This document allows us to give instructions to the Apache web server.
After you open the document or create a new one, you need to define the preferred redirect status code and the new URL to redirect the user to. For example, hereâs how we would create a 301 redirect leading permanently to the landing page of BitDegreeâs website:
Redirect 301 / https://www.bitdegree.org
The .htaccess redirect we created above will redirect the user from any page of the old domain. You can also choose to redirect individual webpages:
Redirect 301 /old-dusty-url.html https://www.bitdegree.org/shiny-new-url.html
Including URL redirects in PHP header function
If you have some basic knowledge of PHP, you can create a simple redirect using its header function. You need to define the new location as you see in the example below:
<?php
header('Location: https://www.bitdegree.org');
exit;
?>
A PHP redirect is a 302 redirect by default. This is okay if you only need a temporary redirect. If you do need it to stay permanently, add a parameter true
and the new status code 301
after the new location:
header('Location: https://www.bitdegree.org', true, 301);
Using url.redirect in Lighttpd
If you are using the Lighttpd web server, there are two steps you need to take:
- Import the
mode_redirect
module - Use
url.redirect
to define the target URL
server.modules = (
"mod_redirect"
)
$HTTP["host"] =~ "^(www\.)?the-old-dusty-website.com$" {
url.redirect = (
"^/(.*)$" => "https://www.bit-degree.org/$1",
)
}
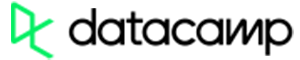
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
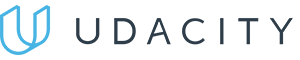
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
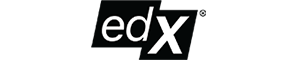
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
The scheme variable in Nginx
If you are using the Nginx web server, the simplest way to create a URL redirect is by using the return
statement:
return 301 https://www.bitdegree.org$request_uri;
This code line should be placed in the server
block:
server {
listen 80;
listen [::]:80;
hostname bitdegree.org www.bitdegree.org;
return 301 https://www.bitdegree.org$request_uri;
}
The redirect function in Flask
If you code in Python and use the Flask framework, you can create a URL redirect by defining a route with the redirect
function. It will work on all the subpages in the domain.
Just as the PHP redirect, this is a 302 redirect by default. If you need a permanent one, define it before the closing bracket:
@app.route('/notes/<page>')
def thing(page):
return redirect("https://www.bitdegree.org/learn/" + page, code=301)
For the Ruby coders: redirects in Rails
If you code in Ruby and use the Rails framework, you can use the redirect_to
method to make a URL redirect:
redirect_to "https://www.bitdegree.org"
Just like before, you will get a 302 redirect by default. To change it, modify the redirectâs status
:
redirect_to "https://www.bitdegree.org", status: 301
redirect_to "https://www.bitdegree.org", status: :moved_permanently
redirect_to "https://www.bitdegree.org", status: 303
redirect_to "https://www.bitdegree.org", status: :see_other
How to redirect a URL on the client side
The browser itself can also redirect the user by following the instructions in the HTML document. There are two ways to do this: a meta redirect and a JavaScript redirect.
Meta redirects: with or without delays?
A simple HTML meta redirect only requires one line of code, placed in the head section on the document. You need to define the time for the delay and the new URL for the redirect to lead to:
<meta http-equiv="refresh" content="5; URL=https://www.bitdegree.org/" />
The unitless number you define (5
in the example) represents seconds. If you wish for the redirect to be applied immediately, specify the time as 0. You can use the delay time to display a message for the user (e.g., explain why the old URL is no longer valid) or include a clickable link:
<head>
<meta http-equiv="refresh" content="5; URL=https://www.bitdegree.org/" />
</head>
<body>
<p>If you are not redirected in five seconds, <a href="https://www.bitdegree.org/">click here</a>.</p>
</body>
Note: if you choose to delay your URL redirect, specify a long enough time for the user to be able to click Back (at least three seconds).
Using the JavaScript window.location object
To create a URL redirect using JavaScript, you will need to work with the Browser Object Model (BOM). It allows JS to work with the browser, which is necessary for a client-side redirect. The simplest way to lead your user to a new URL looks like this:
window.location = "https://www.bitdegree.org"
The window
part can easily be skipped without changing the result:
location = "https://www.bitdegree.org"
While client-side redirects cannot send status codes, most programmers have noticed that search engines treat JavaScript redirects like 301 redirects. However, they will not work for browsers that donât support JS.
Tip: using Wordpress? Redirect easily by using a plugin. Weâd recommend Redirection which is free and works with all Wordpress versions since 4.8.