TL;DR â MongoDB allows you to manage large databases, namely with different data types, faster and easier when compared to relational database management systems such as MySQL.
Contents
What is MongoDB?
MongoDB is an open-source NoSQL database management system that you can use for big data transactions and highly scalable model operations.
It is a non-relational DBSM that doesnât store its data in tables like relational DBMS such as MySQL or SQLite. Instead, MongoDB stores all data in documents known as records. They use a JSON-like syntax.
As a result, MongoDB is better for handling a variety of data types in large amounts. You can easily create documents and collections that do not have to follow a predefined structure. Using a document-based database is a solid option for people that need their database to be highly-scalable and fast.
How to start MongoDB?
The following section of this MongoDB tutorial will explain how to download MongoDB, install and configure it, create a database or a collection, insert a document, and delete a database.
Installation process
The first step of learning how to use MongoDB is downloading it from its official page. Choose Try Free, click Server, select your operating system, and click Download. Double click on the downloaded MSI package (if you are using Windows) and go through the installation process.
Configuration
Once the installation is finished, you need to continue with the configuration process. In the location where you installed MongoDB, you need to create two folders: log and data. Inside the data folder, you need to create a db folder as well. This location is the place for all the data you will store.
Then, run the Command and Prompt window as an administrator and write the following command:
C:\WINDOWS\system32>cd..
C:\Windows>cd..
C:\cd Program Files\MongoDB\Server\3.4\bin
C:\Program Files\MongoDB\Server\3.4\bin
After that, you need to access the bin folder which might be different considering the location you chose during the installation.
Now, run the following command:
mongod --directoryperdb --dbpath "C:\Program Files\MongoDB\data\db" --logpath "C:\Program Files\MongoDB\log\mongo.log" --logappend --rest --install
This command configures the data and log folders while also setting MongoDB as a service.
Finally, type the code below to start MongoDB.
net start MongoDB
There will be a message that says MongoDB service was started successfully. Then, you can enter the MongoDB shell. Repeat this command again:
C:\WINDOWS\system32>cd..
C:\Windows>cd..
C:\Program Files\MongoDB\Server\3.4\bin
C:\Program Files\MongoDB\Server\3.4\bin
Now, you can create a database. The steps for this task are in the following section.
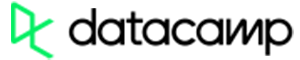
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
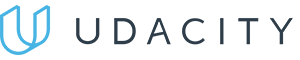
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
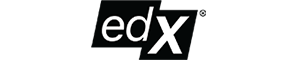
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
How to create a database in MongoDB?
With the last code from the previous section of our MongoDB tutorial, you opened the shell. To run it, type this:
mongo
Now, you can create and name a database:
use the_database_name
Note: once you create a database, it automatically launches. Therefore, this code can be used to run an existing database.
To see if youâre on the right database, you can just type db
as a command. Additionally, you can use the command line show db
to list all the existing databases with their sizes. For instance:
> show dbs
Admin 0.000GB
Local 0.000GB
You wonât see databases on this list if they do not have any documents.
How to create a collection and document in MongoDB?
To create a collection and a document, use the command line below. For example, letâs create a collection named commentators.
> db.commentators.insert({
name: "Samuel",
age: 27})
The document in the example above has name and age alongside each of their values. If you succeed, the command prompt will return:
WriteResult({ "nInserted" : 1 })
Now that you have inserted a document, the next time you use show dbs command line, your database will be displayed on the list.
You can also create a collection without inserting any documents if you want to apply a cap. Below is a list of parameters you can use:
- Capped: set it to
true
if you want to limit the number of entries allowed in the collection. You must state the values for size and max as well. - Size: it dictates the maximum size (in megabytes) allowed for a collection.
- Max: it shows the maximum number of documents allowed in a collection.
- AutoIndexId: setting this parameter to
true
will automatically create the tag_id
for indexing.
Note: the size parameter is mandatory even if you include the maximum number of documents. If the collection exceeds the allocated space, older documents will be removed to make space for new ones.
Here is the syntax for creating a collection without documents:
db.createCollection("log", { capped : true, size : 5555, max : 2000 } )
How to delete a database in MongoDB?
To delete (or drop) the current database, simply type the command line below.
db.dropDatabase()
If you want to drop a different database than the one youâre currently in, you need to run that database.
Use the command show dbs
to show the list of existing databases. Then, type in use the_database_name
.
Once youâre inside the database, use the same command line:
db.dropDatabase()
You can check whether the database is actually deleted by running the show dbs
command again.
MongoDB tutorial: useful tips
- If you just want to drop a collection and not the database, you can use the command line
db.the_collection_name.drop()
. - Deleting the collection will also drop the indexing and the documents inside it. If you prefer to keep the index intact, use the
command db.the_collection_name.remove(delete_criteria)
. - To verify whether a document has been successfully inserted, you can use the command
db.the_collection_name.find()
.