TL;DR – C# lists hold a collection of variables that have the same type.
Contents
What C# List<T> Is
C# specifies the type of elements in a collection between the angle brackets. C# List<T>
refers to a strongly typed list, containing sequences of elements accessible via index.
Note: the C# List<T> is a generic class, declared in the System.Collections.Generic namespace.
To access the List<T>
class, you have to import this namespace to your project by writing using System.Collections.Generic;
.
Follow this code example to create C# List<T>
:
using System;
using System.Collections.Generic;
public class ListExample
{
public static void Main(string[] args)
{
List<string> names = new List<string>()
{"Marge", "Homer"};
foreach (string name in names)
{
Console.WriteLine(name);
}
}
}
The code above creates a list, containing two names.
Manipulating the Length of Lists
It is possible to modify the C# list length. New elements can be added to a collection. The existing elements can be removed as well.
using System;
using System.Collections.Generic;
public class ListExample
{
public static void Main(string[] args)
{
List<string> names = new List<string>()
{"Marge", "Homer"};
names.Add("Lisa");
names.Add("Bart");
names.Remove("Homer");
foreach (var name in names)
{
Console.WriteLine(name);
}
}
}
This type of list also permits accessing elements according to a specified index in the [
and ]
tokens.
using System;
using System.Collections.Generic;
public class ListExample
{
public static void Main(string[] args)
{
List<string> names = new List<string>()
{"Marge", "Homer"};
names.Add("Lisa");
names.Add("Bart");
names.Remove("Homer");
Console.WriteLine(names[1]);
}
}
Remember: in C#, indices begin at 0.
To check the C# list length by counting each element, it is necessary to use the Count
property. The example below counts the number of elements the previously generated list contains:
using System;
using System.Collections.Generic;
public class ListExample
{
public static void Main(string[] args)
{
List<string> names = new List<string>()
{"Marge", "Homer"};
names.Add("Lisa");
names.Add("Bart");
names.Remove("Homer");
Console.WriteLine(names.Count);
}
}
Therefore, the Count
property determines the actual number of elements in a list. However, the Capacity
can set the maximum C# list size. By adding the following code after a generated list, C# will output the maximum number of elements:
using System;
using System.Collections.Generic;
public class ListExample
{
public static void Main(string[] args)
{
List<string> names = new List<string>()
{"Marge", "Homer"};
names.Add("Lisa");
names.Add("Bart");
names.Remove("Homer");
Console.WriteLine("Size of the list = " + names.Capacity);
}
}
Finding Objects in C# List<T>
A list in C# can contain hundreds or thousands of elements. Therefore, it becomes difficult to find specific elements when necessary. The best option is the IndexOf
function which finds elements and returns its index.
The code example below retrieves the index of a specified element. In cases when an item is not found, C# returns -1
:
using System;
using System.Collections.Generic;
public class ListExample
{
public static void Main(string[] args)
{
List<string> names = new List<string>()
{"Marge", "Homer", "Lisa"};
var index = names.IndexOf("Lisa");
if (index > -1)
Console.WriteLine("The name {0} is at index {1}", names[index], index);
var notFound = names.IndexOf("Not Found");
Console.WriteLine("If an item is not found, IndexOf will return {0}", notFound);
}
}
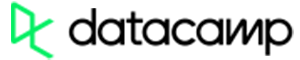
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
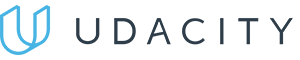
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
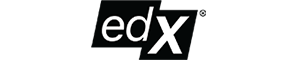
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Sorting Lists
C# list sorting is done with the Sort
method. It arranges all of the elements according to a specified or a default IComparer<T>
.
The following code example sorts the names in alphabetical order and turns them to all caps:
using System;
using System.Collections.Generic;
public class ListExample
{
public static void Main(string[] args)
{
List<string> names = new List<string>()
{"Marge", "Homer", "Lisa"};
names.Sort();
foreach (var name in names)
{
Console.WriteLine("Hello {0}!", name.ToUpper());
}
}
}
There are four options for C# list sorting:
Sort(Comparison<T>)
: arranges all the elements in a list according to the indicatedComparison<T>
.Sort(Int32, Int32, IComparer<T>)
: arranges the elements in a range of elements inList<T>
using the indicated comparer.Sort()
: arranges elements in the entireList<T>
.Sort(IComparer<T>)
: arranges elements in the wholeList<T>
with the specified comparer.
Linked Lists in C#
C# linked list represents a linear collection of nodes. Every node has an address to the next node and can store data (this is called a singly linked list).
Note: every node inside these lists has LinkedListNode<T> type.
C# LinkedList<T> is a doubly-linked list. It means that every node has two addresses: one links to the previous node, and the other links to the next.
Note: the first node has a prev pointer, leading to null. Similarly, the last pointer leads to null.
Follow this code example to create a C# linked list with strings and manipulate their values:
using System;
using System.Collections.Generic;
public class Example
{
public static void Main()
{
// This code creates the linked list.
string[] words = {"the", "mouse", "stole", "the", "yellow", "cheese"};
LinkedList<string> sentence = new LinkedList<string>(words);
Display(sentence, "The values inside a list:");
Console.WriteLine("sentence.Contains(\"steals\") = {0}", sentence.Contains("steals"));
sentence.AddFirst("today");
Display(sentence, "Test 1: Add 'today' to beginning of the linked list:");
// This code moves the first node to be the last position in the list.
LinkedListNode<string> mark1 = sentence.First;
sentence.RemoveFirst();
sentence.AddLast(mark1);
Display(sentence, "Test 2: Move first node to be last node:");
sentence.RemoveLast();
sentence.AddLast("yesterday");
Display(sentence, "Test 3: Add 'yesterday' as the last node:");
}
private static void Display(LinkedList<string> words, string test)
{
Console.WriteLine(test);
foreach (string word in words)
{
Console.Write(word + " ");
}
Console.WriteLine();
Console.WriteLine();
}
}
C# List Remove: Delete Specified Objects
C# List<T>
has several options for removing items from generated collections.
To get rid of certain elements from the C# List, Remove(T)
method is a practical option. It deletes the first occurrence of the object specified in the parentheses (instead of T).
The following code generates a string list and removes one element from it:
using System;
using System.Collections.Generic;
public class ListExample
{
public static void Main(string[] args)
{
List<string> names = new List<string>();
names.Add("Brad Pitt");
names.Add("Oprah Winfrey");
names.Add("Britney Spears");
names.Add("Will Smith");
names.Add("Ellen DeGeneres");
names.Remove("Brad Pitt");
Console.WriteLine("I would like to meet {0}.", names[0]);
Console.WriteLine("I also like {0} and {1}.", names[2], names[3]);
}
}
C# allows removing an item in a specific index. Indicate the index number and the corresponding item will be deleted. The code below removes an element at index 5:
names.RemoveAt(5);
Another option for deleting objects from C# list: RemoveRange()
method. It has two parameters:
- The first one indicates the index to begin the removal from.
- The second indicates the number of items to delete.
The code below removes 3 items starting at index 1.
names.RemoveRange(1, 3);
C# List: Useful Tips
- The letter T in
List(T)
represents the type of objects added to the collection. Compilers check that new objects would have the correct type. - The C# list can have duplicates.
Capacity
presents results that are bigger or equal toCount
. WhenCount
breaks this rule, the capacity automatically increases by reallocating the internal array before copying the old element and adding the new elements.