TL;DR – C# array refers to a collection of variables that have the same type.
Contents
Creating Arrays in C#
C# arrays hold variables of the same type. When you create an array, you specify the type (for instance, int or string) of data it will accept.
Note: C# arrays can hold any elements, even other arrays.
It is possible to access a specific item in the array by its index. The array elements are kept in a contiguous location.
Tip: the contiguous memory allocation refers to the fact that when a process executes, it requests the necessary memory.
An array is usable only after you make C# declare array with a name and an element type. The second pair of square brackets [ ]
indicates the size of the array.
Note: the index of variables in an array begins at 0.
type[ ] arrayName[]
The most basic type of C# arrays is a single-dimensional array, declared with the following code:
int[] array1 = new int[4];
We have created an array and set its size to 4. However, we did not add any variables to it. You can declare an array by assigning elements to it as well:
int[] array1 = new int[] { 1, 3, 4, 7, 8 };
Previous code examples declared and initialized int type arrays in C#. String arrays can be created by using the following code:
string[] firstString = new String[5];
Now, we declare and initialize a C# string array by adding values to it:
string[] dessert = new string[] {"Cupcake","Cake","Candy"};
Remember: C# completes allocation of the memory for arrays dynamically. Since arrays are objects, the retrieval of C# array length is easy by using the prepared functions.
Initialization of Arrays
To make C# initialize arrays, developers apply the new
keyword. Consider this code:
int[] array1 = new int[6];
C# creates an array and reserves memory space for six integers. However, the initialization process does not end here.
It is important to assign values to the array. The most useful way to create arrays and produce quality code is declaring, allocating, and initializing it with a single line:
int[] array1 = new int[6] { 3, 4, 6, 7, 2};
Every element in an array has a default value which depends on the array type. When declaring an int type, you make C# initialize array elements to 0 value.
Multi-Dimensional Arrays
C# multi-dimensional arrays have multiple rows for storing values. It is possible to make C# declare arrays that have either two or three dimensions.
With the following code, C# creates a two-dimensional array (with [,]
):
int[,] array = new int[5, 3];
You can create three-dimensional arrays using this code:
int[, ,] array1 = new int[4, 2, 3];
Jagged Arrays
C# arrays are jagged when they hold other arrays. The arrays placed in jagged arrays can have different sizes and dimensions.
With the following code, we generate a single-dimensional array with four other single-dimensional int type arrays:
int[][] jaggedArray = new int[4][];
It is crucial to make C# declare arrays and initialize them with the following code:
jaggedArray[0] = new int[4];
jaggedArray[1] = new int[5];
jaggedArray[2] = new int[3];
We created three single-dimensional arrays: the first one contains 4 integers, the second contains 5 integers, and the last one has 3 integers.
You can make C# initialize arrays without setting their size as well. Then, you have to include their element values by using the initializers:
jaggedArray[0] = new int[] { 7, 2, 5, 3, 8 };
jaggedArray[1] = new int[] { 4, 5, 7, 8 };
jaggedArray[2] = new int[] { 14, 18 };
Remember: the elements of jagged C# arrays are reference types, and their default value is null.
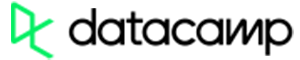
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
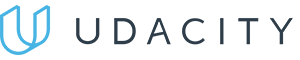
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
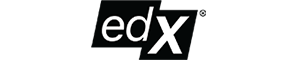
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Accessing and Adding Values to Arrays
You can make C# add to array new values even after declaration and initialization processes by indicating a specific index.
First, we declare and initialize an array:
int[] array1 = new int[6];
Then, we set a value of 12 for an array in its index 0:
intArray[0] = 12;
The following code assigns a value of 25 for an array in its index 1:
intArray[1] = 25;
We assign a value of 32 for an array in its index 2:
intArray[2] = 32;
To access a specific value in an array, you should indicate the exact index:
intArray[0]; //returns 12
Methods and Properties for Arrays
Here is a list of the most popular methods applied to arrays C#:
Method | Description |
---|---|
GetLength(int dimension) | Retrieves the number of elements in the indicated dimension. |
GetLowerBound(int dimension) | Retrieves the lowest index of the indicated dimension. |
GetUpperBound(int dimension) | Retrieves the highest index of the indicated dimension. |
GetValue(int index) | Retrieves the value at the indicated index. |
ForEach(int32) | Retrieves the 32-bit integer, revealing the number of elements in the indicated dimension. |
Resize(T[], Int32) | Changes the size of a specified single-dimensional array. |
A useful tip is to explain how converting a C# byte array to string works:
string s = System.Text.Encoding.UTF8.GetString(buffer, 0, buffer.Length);
There is another option which is split into two parts. You have to include this code for this conversion to work:
UTF8Encoding utf8 = new UTF8Encoding(true, true);
Then, you can use the following code to convert C# byte array to string:
String s2 = utf8.GetString(bytes, 0, bytes.Length);
The following table shows the most common properties for arrays:
Property | Description |
---|---|
ICollection.Count | Delivers the number of elements in an array. |
ICollection.IsSynchronized | Delivers a value revealing whether the access to an array is synchronized. |
IsFixedSize | Retrieves a value revealing whether an array has a fixed size. |
Length | Delivers the number of elements in all dimensions of the array. |
Rank | Delivers the number of dimensions of an array. |
IsReadOnly | Delivers a value revealing whether an array is read-only. |
foreach Method
The foreach
is an easy method for processing all elements in an array. It influences the elements from index 0 to -1.
The following code example shows how to use foreach
to print the values in an array to the console:
public class Program
{
public static void Main()
{
int[] numbers = {7, 12, 1, 5, 3, 8, 9, 20, 4};
foreach (int i in numbers)
{
System.Console.Write("{0}", i);
}
}
}
C# Array: Useful Tips
- The number and length of dimensions of C# arrays cannot be modified after they are declared.
- Arrays are great for code optimization because developers can store multiple values in one variable instead of creating multiple variables.
- The square brackets (
[]
) after the element type indicate that it is an array.