TL;DR – C# enum refers to a keyword for indicating an enumeration of integral constants.
Declaring enum: Possible Types and Use
The C# enum
keyword indicates a collection of named integral constants.
Specifying C# enums within a namespace is the best practice as all the classes will be able to access it. It is also possible to place enum
within a struct or a class.
Here is a basic code example, showing the way to declare enums:
enum grades {A, B, C, D, E, F};
Note: by default, C# enum type for elements is int. You can set it to different integral numeric by adding a colon.
The following code reveals the way to manipulate the type of elements:
enum grades : byte{A, B, C, D, E, F};
Here is a list of C# enum types that can replace int:
- sbyte
- byte
- short
- ushort
- int
- uint
- long
- ulong
If you need other options for the conversion of C# enum, string is also a possibility. To convert enums to strings, you should apply the ToString
function:
using System;
public class EnumSample
{
enum Holidays
{
Christmas = 1,
Easter = 2
};
public static void Main()
{
Enum myHolidays = Holidays.Christmas;
Console.WriteLine("The value of this instance is '{0}'", myHolidays.ToString());
}
}
Note: you cannot set an enum to string as enums can only have integers. The conversion above simply converts an already existing enum into a string.
It is set that every enumeration begins at 0. With each element, the value increases by 1. However, it is possible to manipulate this default rule by specifying a value to elements in the set. In the C# enum example below, we used an initializer to assign a value to the elements.
enum grades {A=1, B, C, D, E, F};
You can use functions to make your code perform certain functions. For instance, the following code prints out all the elements in an enum:
using System;
public class EnumSample
{
enum Holidays
{
Thanksgiving = 1,
Christmas = 2,
Easter = 3
};
public static void Main()
{
foreach (var item in Enum.GetNames(typeof (Holidays)))
{
Console.WriteLine(item);
}
}
}
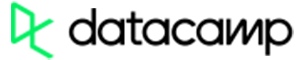
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
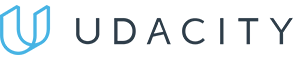
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
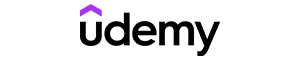
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Adding Flags to C# Enum
There are two C# enum types: simple and flag. The flag type is for supporting bitwise operations with the enum values.
The [Flag]
attribute is for representing a set of possible values and not a single value. Therefore, such sets are often used for bitwise operators.
The following C# enum example shows how to assign a flag on an enum by applying the bitwise OR
operator:
using System;
public class Program
{
enum Roommates
{
Josh = 1,
Carol = 2,
Christine = 4,
Paul = 8
}
[Flags]
enum RoommatesPav
{
Josh = 1,
Carol = 2,
Christine = 4,
Paul = 8
}
public static void Main()
{
var str1 = (Roommates.Josh | Roommates.Paul).ToString();
var str2 = (RoommatesPav.Josh | RoommatesPav.Paul).ToString();
Console.WriteLine(str1);
Console.WriteLine(str2);
}
}
C# enum: Useful Tips
- C# enums define integers more consistently and clearly. There are fewer chances that your code will contain invalid constants.
- It is usually better to represent items with
enum
when their order in an enumeration is important. - Be careful not to add the same values to different elements represented by
enum
. It can cause inconsistencies in your code and render unwanted results.