TL;DR ā Converting string to int and vice-versa in C++ can be done in two ways, depending on the C++ version you're using.
Contents
Converting a String to an Integer
There are two methods of converting a string to an integer. Which one you should use depends on the version of your C++: whether itās C++11 and above, or an earlier version of the language.
C++03 and Earlier
For the earlier versions of C++, weāre going to use the stringstream class to perform input-output operations on the strings.
Letās say we have a string called s
with a value of 999
, and we want to convert that string into an integer called x
. Hereās how to do it:
#include <iostream>
#include <sstream>
using namespace std;
int main() {
string s = "999";
stringstream degree(s);
int x = 0;
degree >> x;
cout << "Value of x: " << x;
}
In the example above, we declare degree
as the stringstream object that acts as the intermediary and holds the value of the string. Then, by entering degree >> x
, we extract the value out of the object and store it as integer x
.
Finally, use the cout
function to display the result. If you use the code above properly, your output should look like this:
Value of x: 999
C++11 and Above
The C++11 update came with the new stoi()
function which makes converting string to int much easier. In the example below, weāre converting a string named pp
into an integer called num1
:
#include <iostream>
#include <string>
using namespace std;
int main() {
string pp = "123";
int num1 = stoi(pp);
cout << "stoi(\"" << pp << "\") is " << num1 << '\n';
}
And hereās the output:
stoi("123") is 123
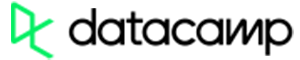
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
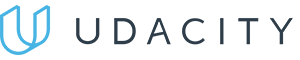
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
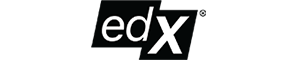
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Converting an Integer to a String
Just like before, the method of converting an int to a string in earlier versions of C++ differs from the one used in C++11 and above.
Up to C++03
Users of this version can use the std::stringstream
class from the C++ standard library. The following example converts the integer x
into a string named out_string
:
#include <sstream>
int main() {
int x = 123;
std::string out_string;
std::stringstream ss;
ss << x;
out_string = ss.str();
}
Hereās what happens when you use the code above:
- Before converting the integer
x
, a string is declared through thestd::string
class. - The stringstream object called
ss
is declared. - An operator is used to insert the data from integer
x
to the stringstream object. - The
str()
function returns theout_string
with a value of123
.
Since C++11
For newer versions of C++, weāre going to use the std::to_string()
class. Take a look at the example below, where integer n
is converted into a string called str
:
#include <iostream>
#include <string>
int main() {
int n = 123;
std::string str = std::to_string(n);
std::cout << str << "\n";
}
As you can see, the new string class converts a string into an integer directly without having to use a stringstream object as the intermediary.
String to Int C++: Useful Tips
- You can end the code with
\n
or\endl
. The former inserts a new line, while the latter inserts a new line and flushes the stream. - If you want to redeclare the same stringstream with the same name, you can use
str()
to empty the stringstream.