TL;DR â C++ map is an associative container used for storing and sorting elements.
Contents
What is a C++ Map?
C++ map is an associative container that is used to store and sort elements in an orderly fashion. Itâs a part of the C++ Standard Template Library. Using the map, you can effortlessly search for elements based on their keys.
Keys and values are essential to a C++ map. This is because elements are key-value pairs that follow a specific sequence. You can add or delete keys, but you canât alter them. Values, on the contrary, can be changed.
Note: since the keys are unique, no two values can have the same keys.
C++ Map Syntax
The map syntax parameters are:
-
Key
specifies the keys that are going to be saved in the map. -
T
represents the content that associates with the key. -
Compare
is a binary predicate. It takes two keys as arguments and returns a bool. -
Alloc
defines the storage allocation model. Usually it goes by the default value.
template < class Key, // map::key_type
class T, // map::mapped_type
class Compare = less<Key>, // map::key_compare
class Alloc = allocator<pair<const Key,T> > // map::allocator_type
> class map;
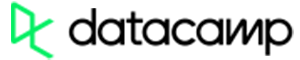
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
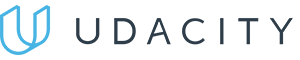
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
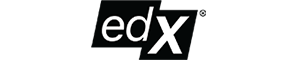
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Creating a Map in C++
When it comes to making a map, remember that the keys and their corresponding values always come in pairs. It wonât work if you only insert the key or just the value.
You can easily create a map with:
typedef pair<const Key, T> value_type;
template<
class Key,
class T,
class Compare = std::less<Key>,
class Allocator = std::allocator<std::pair<const Key, T> >
> class map;
namespace pmr {
template <class Key, class T, class Compare = std::less<Key>>
using map = std::map<Key, T, Compare,
std::pmr::polymorphic_allocator<std::pair<const Key,T>>>
}
Basic C++ Map Functions
Just like any other programming language, C++ needs functions for the programs to work. Functions are specific instructions or blocks of code that tell how, what, where, and when a task should be done. Same goes with the map in C++.
Here are some basic functions related to C++ maps that can help you get started:
Function | Definition |
---|---|
begin() | Returns a bidirectional iterator (the pointer) to the first element of the map container. It should run in constant time. |
end() | Returns the bidirectional iterator just past the end of the map. Youâll have to decrement the iterator before you can access the last element of the container. |
empty() | Checks if the map container is empty or not. Returns TRUE if the container has no elements and FALSE if otherwise. |
insert({key, element}) | Adds some data or an element with a particular key into the map. |
find(key) | Runs in logarithmic time and returns an iterator to where the key is present in the map. If the key is not found, the iterator is returned to the end of the map. |
size() | Returns the total amount of elements present in the map container. |
erase() | eEliminates keys and elements at any given position or range in the map. |
clear() | Runs in linear time, this function deletes all elements from the map and leaves 0 as its size. |
C++ Map: Useful Tips
- C++ Maps let you store data with any type of key, not just a numerical key.
- To dynamically handle its storage needs, the map uses an allocator object.
- Maps are also implemented as binary search trees.