TL;DR – C++ strings are objects that represent sequences of characters. They're considered the driving force of coding in C++.
Contents
What are C++ Strings?
A C++ string is a one-dimensional array of characters that ends with a null character \0. You define a string with the following syntax:
using namespace std;
int main() {
char str[] = "C++";
}
Here is a basic example of a C++ string:
using namespace std;
int main () {
char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
}
In this case, a string consists of the different letters that make up the word 'Hello'. If you initialize the array, that is exactly the output you will get.
String Length
To find the length of a string in C++, you can use the size()
or length()
functions. Below is an example of how to accomplish just that.
#include <iostream>
using namespace std;
int main() {
string str = "C++ Programming";
// str.length() can also be used
cout << "String Length = " << str.size();
}
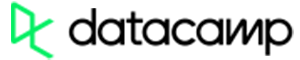
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
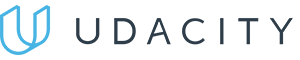
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
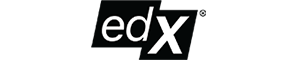
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Defining a Class in C++
C++ is an object-oriented programming language. To learn how to create a string as an object that holds several strings, look at the example below:
#include <iostream>
using namespace std;
int main() {
// String object declared
string str;
cout << "Enter a string: ";
getline(cin, str);
cout << "You entered: " << str << endl;
}
Once a string is declared, it’s asked by the user. Using this method, there’s no length cap.
C++ String: Useful Tips
- Use
c_str()
instead oftoCharArray()
when you need to call thesend()
function. This will prevent duplication of the string. - Pass string objects by as references rather than values. The code will be much more efficient as it won’t copy a string, but just reference it.
- Initialize strings from Flash to save RAM by only loading the data when the string is loaded. Simply add 'F' before your body.