TL;DR – The getline C++ command allows you to process multiple strings at once.
Contents
What is Getline in C++?
The getline()
command reads the space character of the code you input by naming the variable and the size of the variable in the command. Use it when you intend to take input strings with spaces between them or process multiple strings at once.
You can find this command in the <string> header. It extracts the input stream’s characters and attaches them to the string continuously until it reaches the delimiting point.
Getline C++ Syntax
The syntax for getline C++is quite straightforward:
is
is an object of theistream
class which tells the function of the stream the input that needs to be read.str
is the string object that stores the input after the reading process of the stream is finished.delim
defines the delimitation character which commands the function to stop processing the input. The reading process will stop once this command is read by the written code.
istream & getline (istream & is, string & str, char delim);
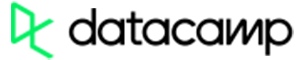
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
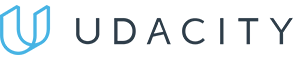
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
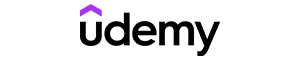
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Most Common C++ Getline Application
A common application of C++ getline is to read a string from the input stream. You can use it for a simple, practical purpose of displaying information:
#include <iostream>
using namespace std;
//macro definitions for max variable length
#define MAX_NAME_LENGTH 50
#define MAX_ADDRESS_LENGTH 100
#define MAX_ABOUT_LENGTH 200
using namespace std;
int main () {
char name[MAX_NAME_LENGTH], address[MAX_ADDRESS_LENGTH],
about[MAX_ABOUT_LENGTH];
cout << "Enter name: ";
cin.getline (name, MAX_NAME_LENGTH);
cout << "Enter address: ";
cin.getline (address, MAX_ADDRESS_LENGTH);
cout << "Enter about yourself (press # to complete): ";
cin.getline (about, MAX_ABOUT_LENGTH, '#'); //# is a delimiter
cout << "\nEntered details are:\n";
cout << "Name: " << name << endl;
cout << "Address: " << address << endl;
cout << "About: " << about << endl;
}
The output of the above code will look like this:
Enter name: Mr. Mike Thomas
Enter address: 101, Phill Tower, N.S., USA
Enter about yourself (press # to complete): Hello there!
I am Mike, a website designer.
My hobbies are creating web applications.#
Entered details are:Name: Mr. Mike Thomas
Address: 101, Phill Tower, N.S., USA
About: Hello there!
I am Mike, a website designer.
My hobbies are creating web applications.
Getline C++: Useful Tips
- You can create a
stop
character in getline to end the input. This character will finish the command and be moved from the input. - Using
std::cin >> var.
beforestd::getline()
can cause problems. As a solution, you can create a stop character as a third argument, allowing C++ getline to continue the reading process. - When using non-processable input in
std::cin
, it will end in a fail state. This can cause a serious problem since all input will be put on hold until thefail
state is fixed. To remove the problematic input, addstd::cin.ignore
. - C++ getline can be set to stop processing the input at a specific character:
std::string temp
(std::cin, temp, '|')