Before we start learning, we should first answer the most basic question: what is jQuery? To put it simply, it is a JavaScript library based on a simple principle: write less, do more. This library simplifies JavaScript event handling, traversing HTML documents, AJAX-based interactions, and animations.
To learn jQuery and start using it in your daily coding, you only need basic knowledge of CSS, HTML, and JavaScript. This makes jQuery fairly beginner-friendly. In the following sections, we will explain what is jQuery used for, so you can see how you as a developer can benefit from it.
We will also introduce the standard jQuery syntax, and present you with a few simple jQuery examples to illustrate the basic usage.
Contents
What Can jQuery Do?
To properly explain what is jQuery used for, we should first note that its main advantage is the ability to streamline the development of various features that would otherwise require extensive work with JavaScript to implement.
Another important feature is its compatibility. The team behind jQuery has done a great job addressing the cross-browser issues, and the library will work the same on all major browsers.
To sum up, what is jQuery used for, we should pay attention to the following supported features:
- HTML event methods
- HTML/DOM manipulation
- CSS manipulation
- AJAX
- Utilities
- Effects and animations
In this tutorial, we will talk about each of these features briefly so you are able to get the main idea before you learn jQuery. Each of them will be thoroughly explained in the upcoming tutorials.
Syntax
Basically, jQuery syntax for any statement follows the same pattern. An item from the HTML DOM is selected, and action in the form of a method or function is applied:
$(selector).methodOrFunction();
As you can see, the jQuery syntax is not complicated at all. All statements come from a basic template for applying functions and methods to selected HTML elements and their attributes. This method can be easily modified depending on the selector and the action, which is the applied method or function.
In various Internet tutorials you can find the methods and functions defined in different manners: method, .method, method() or .method(). We will be following this last format to comply with the official jQuery documentation. If you see a term written without the period and parenthesis, you can be sure it's not a method or function: it's either a event or a property.
Note: don't get puzzled if you see method names without a period or parentheses in other websites: as there are no official rules, the usage of term seems to vary across sources.
Document Ready Event
Before jQuery is applied, it is important to make sure that all HTML elements have been loaded, and the CSS is fully computed. These conditions are necessary for jQuery to be applied properly.
To make sure that is the case, we place all jQuery inside the document ready event. This means that the statements inside the block of code will only be applied once the web page has finished loading. Here's an example that you can copy and paste into your code to get started with applying jQuery methods:
There is one more way of writing the document ready event. It is shorter and therefore often used for convenience, which is important as you learn jQuery:
As you review the jQuery examples above, keep in mind that while waiting for the page to load fully is the way that jQuery is usually applied, there are also instances when it is preferable to apply it before the page is loaded. A good example could be wishing to hide a particular element, or get the size of an element or image which has not loaded yet.
HTML Event Methods
Using jQuery library also provides ways to simplify event handling. The methods it provides are short and simple. Using them allows you to:
- Add, change and remove event handlers on specified elements.
- Trigger various events via code.
- React to events in more specific, complex ways.
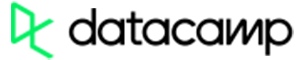
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
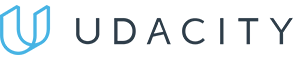
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
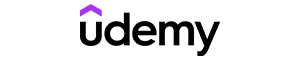
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
HTML DOM And CSS Manipulation
jQuery also provides methods that can be used to access the HTML DOM and CSS in different ways. These manipulation methods allow you to:
- Retrieve DOM information about the HTML elements, their attributes, and the values of attributes.
- Get the values of computed CSS properties which belong to a specified set of elements.
- Remove and add HTML elements in different ways based on the method used.
- Modify the values of CSS properties of HTML elements.
AJAX
AJAX, simply put, is a way of using JavaScript that allows you to refresh a part of the page without needing to refresh all of it. The jQuery library introduces a full suite of AJAX-related methods to make its implementation easier.
The AJAX-based methods in the jQuery library allow you to:
- Register, start, stop, and follow AJAX events.
- Encode elements as strings for submission.
- Load data from the server via asynchronous requests.
Utility Methods
There are also a few utility-based methods you may find useful. As you learn jQuery, you will be able to:
- Execute JavaScript globally.
- Examine arguments to determine their data type.
- Parse strings for various purposes.
- Clear the queue or move on to the following function in it.
Effects and Animations
When covering what is jQuery used for, we cannot skip the variety of methods for implementing animations and effects it provides. Using them, you can:
- Perform custom animations via modifying a set of CSS properties.
- Animate and set the opacity or position of various elements.
- Disable and enable animations globally.
- Create and manipulate a queue of animations.