Using jQuery helps us access and handle files way more easily than by using pure JavaScript. DOM traversal methods are among those used the most often. They allow the developer to select HTML elements in a document. However, sometimes too many matches get returned. In these cases, we use jQuery filters.
By filtering the matched elements, you can only select those that check out with a chosen condition. This makes moving through the DOM element tree much more manageable and gives you more control. In this tutorial, we will present different filtering methods and provide you with useful code examples.
Contents
jQuery Filter: Main Tips
- In jQuery, traversing refers to moving through the DOM tree to access certain HTML elements.
- You can also filter the selections using specialized jQuery methods.
- Using jQuery filters, you can match methods against selectors or functions.
Methods to Choose From
The jQuery library provides a few methods for the purpose of filtering:
.first()
returns the first of the specified elements..last()
returns the last of the specified elements..eq()
returns an element with a specific index from the specified elements..filter()
returns elements that meet a certain condition from the specified elements..not()
returns elements that do not meet a certain condition from the specified elements.
We will now review each of these filters jQuery and provide useful code examples.
The .first()
method returns the element that is first index-wise from the selection made via the selector:
$(selector).first()
The example below illustrates the usage of this method. You can see the first one of five elements is selected and highlighted in red:
The .last()
method works in an opposite way. It returns the last element from the selection:
$(selector).last()
See the example below. The same selection of elements is used, but the result is different:
The .eq()
method returns an element with a specific index number from the selection:
$(selector).eq(index | indexFromEnd)
The index is a simple numerical value in this context. The indexFromEnd is also a numerical value and counts the index from the very end. The key difference between index and indexFromEnd is that the latter is written as a negative value.
View the example below. Notice the value that has been defined in the search, and try changing it in the code editor to see the results changing:
The jQuery .filter()
method matches selected elements against a certain condition and returns those that meet it. The .not()
method matches the selection against a condition as well, but returns those that do not meet it.
Both of these filters jQuery offers use a similar syntax pattern:
$(selector).filter | not(selector | function | elements | selection)
See both of them in the same example to better grasp the difference:
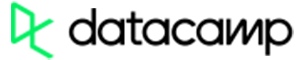
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
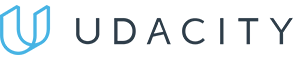
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
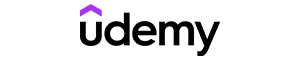
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
Filtering Against Functions
jQuery filters allows us to match a method against a function instead of a specified selector. Let's take a look at a simple example first:
$("li").filter((index) => {
return index % 2 == 1;
}).css("background-color", "red");
$("li").not((index) => {
return index == 3;
}).css("background-color", "blue");
The function that the selection is matched against returns a boolean value for each element in the selection, based on whether they meet the condition. An important aspect of the function is the return statement which sets the condition for which elements will get selected.
A basic template for a jQuery .filter()
function could look like this:
$(selector).filter | not(function( index ){ return comparison; })
In the case of such syntax, the elements that are selected from the selection and meet the condition set by the comparison operator are returned as true.
There is also an alternative way to achieve the same results using jQuery .filter()
function:
$(selector).filter | not(function( index ){ if(comparison) { return true; } })
Using Index and Modulus
When filtering, you can access the index object and select elements with a specified index inside the selector.
You can also select every second, third, or another element by using the modulus operator (%
). For example, return index % 2 === 1;
will return all the elements that have a remainder of 1 when you divide them by 2. The result is every second element: 1, 3, 5, 7, and so on.
Note: In the filter jQuery function, this refers to the currently selected DOM element.
jQuery Filter: Summary
- jQuery traversing allows you to select and access certain HTML elements.
- You may use special methods to filter jQuery selections according to your needs.
- jQuery filters allow you to match a method against either a selector or a function.