For more efficient user experience, HTTP asynchronous requests are preferred. By applying jQuery AJAX, the website performance is not disturbed by initiated requests and works as usual. Synchronous requests, on the other hand, might lead to unresponsive user experience.
With the AJAX jQuery function, a request can be sent to a string, representing an URL. It is possible to use jQuery.ajax() function to send requests. However, sometimes it is more efficient to apply jQuery AJAX get or load methods instead.
Contents
jQuery AJAX: Main Tips
- The jQuery library provides methods to make implementation of AJAX more convenient.
- AJAX is short for Asynchronous JavaScript And XML. Read more about it in this AJAX introduction tutorial.
- This tutorial is about AJAX in the context of jQuery and its methods.
Simpler Usage of AJAX with jQuery
AJAX loads data on a certain element of a page without reloading the whole page.
Notable examples of AJAX implementation include Google Maps and search engine's suggestion system, Twitter, Youtube, and quite a few other major virtual platforms. jQuery provides a very convenient way of implementing it with relatively minimal knowledge.
Main Methods Explained
To handle AJAX jQuery has a few handy methods:
.load()
: load data from the server and place it in the selected element..get()
: request data from the server via an HTTP GET request..post()
: request data from the server via an HTTP POST request.
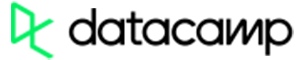
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
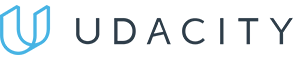
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
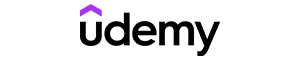
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
- Free certificates of completion
.load()
.load()
is a powerful and convenient method used to load data and display it. The syntax for this method is fairly straightforward as well:
$(selector).load(URL,[data],[callback]);
It accepts three arguments:
- URL: the URL you would like to load.
- data: a plain object or string, sent with the request to the server.
- callback: the function to execute upon request completion.
This method is also the simplest one for fetching data. Upon a successful response, it simply fetches the data and places it in the selected element.
Here is a simple example:
$("#ajaxLoad").load("ajax/document.html #target");
The code snippet above will simply find the file in the ajax folder, which would need to be in the same document as the one from which .load()
is executed, and in the ajax folder, the file document.html would be loaded.
By using a selector next to the address, we specify that only elements with the ID target
will be loaded.
Now, we could provide a callback function to alert us when the method has been executed:
$("#ajaxLoad").load("ajax/document.html #target", function() {
alert("The target element has been loaded!");
});
Method Data Parameter
The second parameter of the .load()
method accepts two kinds of values:
- String
- Plain Object
The important thing about these two is that strings make the request of .load()
to use the HTTP GET method.
With the plain object, the request will be made using the HTTP POST method.
Have a look at this example to understand the usage of this parameter as a plain object in the .load()
method:
$("#feed" ).load( "feed.php", { limit: 20 }, function() {
alert( "The 20 latest entries have been loaded");
});
The above example passes the plain object limit with the value of 20 to specify the number of entries to be loaded. Plain objects are distinguished by being wrapped in curly brackets {}
.
Generally, plain objects are used to send data which can then be used as arguments.
Now, let's take a look at a .load()
method with the data parameter having a string value:
$("#books").load("books.php", "book=lordoftherings");
In this case, the query string we entered is turned into ?book=lordoftherings
and appended to the link. It would result in the corresponding data being loaded.
.get()
The .get()
method is used for performing a HTTP GET request. It follows a specific syntax:
$(selector).get(url,[data],[success],[dataType])
The parameters it accepts are as such:
- URL: the URL you would like to load.
- data: a plain object or string, sent with the request to the server.
- success: the callback function executed on successful request.
- dataType: the type of data expected to be returned from the server.
Additionally, this function is a shorthand. It is the same as:
$.ajax({
url: "url",
data: "data" | {data},
success: function(){/* executable code */},
dataType: "dataType"
});
Its usage is rather similar to .load()
, but can load more specific data, and only via GET requests (which cannot load data onto the server).
Here are some examples of how it could be used to pass arguments and get data:
// returns the entries.php document
$.get( "entries.php" );
// returns the entries.php document while passing two arguments with the request
$.get( "entries.php", { id: "2", name: "Bob" } );
// returns the entries.php document while passing an array argument with two items inside with the request
$.get( "entries.php", { "choices[]": ["Bob", "Beth"] } );
Additionally, you can specify a callback function to be executed if the request is successful, and expected data type of the returned data.
Here is a basic example how:
$.get( "entries.php", function( data ) {
$( ".load-data" )
.append( "ID: " + data.id ) // ID of the entry
.append( "Name: " + data.name ) // name of the entry
}, "json" );
.post()
The AJAX .post()
method is used for performing an HTTP POST request. The syntax for this method is:
$(selector).post(url,[data],[success],[dataType])
It accepts the following parameters:
- URL: the URL you would like to load.
- data: a plain object or string, sent with the request to the server.
- success: the callback function executed on successful request.
- dataType: the type of data expected to be returned from the server.
Additionally, this function is a shorthand. It is the same as:
$.ajax({
type: "POST",
url: "url",
data: "data" | {data},
success: function(){ /* executable code */ },
dataType: "dataType"
});
Similarly to jQuery AJAX .get()
, .post()
also loads data from the server. In addition to that, it can also upload certain data to the server, which can be very useful when working with forms.
Here are some examples of how AJAX .post()
could be used to pass arguments and get data:
// returns the test.php document
$.post("entries.php");
// returns the test.php document while passing two arguments with the request
$.post("entries.php", { id: "2", name: "Bob" });
// returns the test.php document while passing an array argument with two items inside with the request
$.post( "entries.php", { "choices[]": ["Bob", "Beth"] });
// You can also send form data using this method
$.post("entries.php", $( "#sampleForm" ).serialize());
Additionally, you can specify a callback function to be executed if the request is successful, and the expected data type of the returned data.
This code example illustrates how this is done:
$.post("entries.php", function( data ) {
$( ".load-data" )
console.log( data.id ) // ID of the entry
console.log( data.name ) // name of the entry
}, "json");
jQuery AJAX: Summary
- jQuery offers methods for sending asynchronous requests and improving website performance.
- You can use
.get()
,.post()
,.load()
functions to load or request data from the server.